You need to:
- use
tk.Entry.insert
to add the default text to an Entry
widget
- set the foreground color to 'grey'
- upon the entry getting focus, the default is deleted, and the foreground set to 'black'.
- you type in the text.
- upon pressing return, the value of the entry is extracted, then the entry is reset with the default text in grey.
- exiting the focus also resets the entry to default grey (you may want to choose avoid this as a partial entry will then be deleted if you make the entry lose focus; by clicking outside the box, for instance)
Here is what the code look like
import tkinter as tk
def handle_focus_in(_):
full_name_entry.delete(0, tk.END)
full_name_entry.config(fg='black')
def handle_focus_out(_):
full_name_entry.delete(0, tk.END)
full_name_entry.config(fg='grey')
full_name_entry.insert(0, "Example: Joe Bloggs")
def handle_enter(txt):
print(full_name_entry.get())
handle_focus_out('dummy')
root = tk.Tk()
label = tk.Label(root, text='First and last name:')
label.grid(sticky='e')
full_name_entry = tk.Entry(root, bg='white', width=30, fg='grey')
full_name_entry.grid(row=1, column=1, pady=15, columnspan=2)
full_name_entry.insert(0, "Example: Joe Bloggs")
full_name_entry.bind("<FocusIn>", handle_focus_in)
full_name_entry.bind("<FocusOut>", handle_focus_out)
full_name_entry.bind("<Return>", handle_enter)
root.mainloop()
Here is what it looks upon opening the window:

Upon giving focus to the Entry
widget, the example text is deleted, and the font color changed to black; after filling the entry, the aspect is:
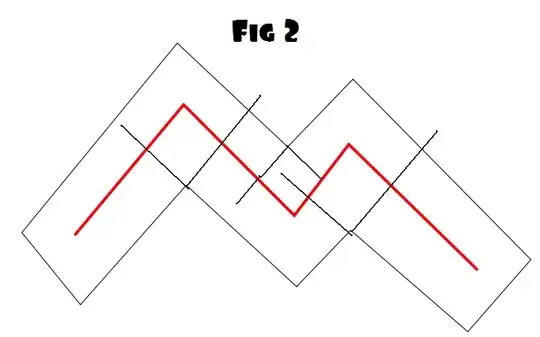