Is it possible to add a background image to the Scaffold's AppBar? I know about sliver but when you scrolldown the image gets hidden and the AppBar changes color right? So I want to know if this is possible and if not, are there any existing workarounds? Thanks!
Asked
Active
Viewed 3.7k times
7 Answers
41
Rather than using a Stack
widget like Zulfiqar did, pass your background image in the flexibleSpace
argument of the AppBar
widget instead:
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('App Bar!'),
flexibleSpace: Image(
image: AssetImage('assets/image.png'),
fit: BoxFit.cover,
),
backgroundColor: Colors.transparent,
),
body: Container(),
);
}

Hafiz
- 507
- 4
- 11
-
2This won't make the `AppBar` as big as your image, it will clip the image. – Michel Feinstein Feb 11 '20 at 02:00
-
If you want to resize the AppBar for a bigger image or something you can set the height yourself AppBar( toolbarHeight: 100, // default is 56 toolbarOpacity: 0.5, ), – Aditya Mittal Nov 28 '21 at 06:32
-
I also have the clipping problem as @MichelFeinstein had. Is there a solution for this? – Henrique Guimarães Oct 14 '22 at 15:18
-
I solved the clipping by wrapping the Container with Transform.translate and setting an offset of (-20, 0). – Henrique Guimarães Oct 14 '22 at 15:48
14
I've had some problems using iOS with Hafiz Nordin's answer. At iOS the image doesn't cover the complete appbar leaving a small transparent space left.
The solution for me was to use an container with a DecorationImage
instead.
AppBar(
flexibleSpace: Container(
decoration:
BoxDecoration(
image: DecorationImage(
image: AssetImage(),
fit: BoxFit.cover,
),
),
),
backgroundColor: Colors.transparent,
title: Text("App Bar"),
);

Jonas Franz
- 555
- 1
- 8
- 18
10
Widget build(BuildContext context) {
return new Container(
child: new Stack(children: <Widget>[
new Container(
child: new Image.asset('assets/appimage.jpg'),
color: Colors.lightGreen,
),
new Scaffold(
appBar: new AppBar(title: new Text('Hello'),
backgroundColor: Colors.transparent,
elevation: 0.0,
),
backgroundColor: Colors.transparent,
body: new Container(
color: Colors.white,
child: new Center(
child: new Text('Hello how are you?'),),)
)
],),
);
}

Sher Ali
- 5,513
- 2
- 27
- 29
-
Hi Zulfiqar. Is there a solution wherein it's more like in a theme? I want to change all the appBar's background image. Since I have a lot of screens, I don't want to apply changes to all screens. Thank you! – Ny Regency Sep 08 '18 at 14:24
-
1Can't you just create one container widget and use it in all your screens? – Ciprian May 08 '19 at 15:56
7
full example
Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
title: Text('How to Flutter', style: TextStyle(
color: Colors.white,
fontSize: 28
),) ,
elevation: 0,
backgroundColor: Colors.transparent,
),
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('assets/bg.jpg'),
fit: BoxFit.fill
)
),
),
)
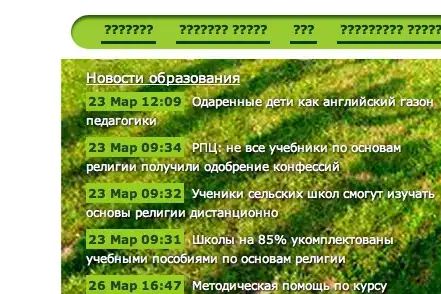

Faiz Ahmad Dae
- 5,653
- 2
- 14
- 19
3
It can be done one of the two ways:
Background image just for Scaffold's appBar
appBar: AppBar(
title: const Text(
"Home Page",
style: TextStyle(color: Colors.white),
),
flexibleSpace: const Image(
image: NetworkImage(
'https://th.bing.com/th/id/OIP.AjOYK61Fo6R4XdkOHnh_UQHaBr?pid=ImgDet&rs=1'),
fit: BoxFit.fill,
),
),
Background image spanning accross body of Scaffold
Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
elevation: 0,
title: const Text(
"Home Page",
style: TextStyle(color: Colors.black),
),
backgroundColor: Colors.transparent,
),
body: Container(
height: 200,
width: double.infinity,
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.fill,
image: NetworkImage(
'https://th.bing.com/th/id/OIP.AjOYK61Fo6R4XdkOHnh_UQHaBr?pid=ImgDet&rs=1'))),
));

krishnaacharyaa
- 14,953
- 4
- 49
- 88
1
appBar: AppBar(
title: Text('Current deals'),
flexibleSpace: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/nav-header.png'),
fit: BoxFit.cover,
),
),
),
),

Girish Chauhan
- 167
- 1
- 4
0
You can use the "extendBodyBehindAppBar: true," property of scaffold to do all the stuff.
Here is an example:
Scaffold(
extendBodyBehindAppBar: true, // <-- Here set is true
appBar: AppBar(
title: Text(
'How to show background Image in appbar',
style: TextStyle(color: Colors.white, fontSize: 28),
),
elevation: 0,
backgroundColor: Colors.transparent,
),
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('assets/background.png'), fit: BoxFit.fill)),
),
);

Shabbir Rajput
- 56
- 5