After researching I found a way that gives me exactly what I needed, this is what I got:
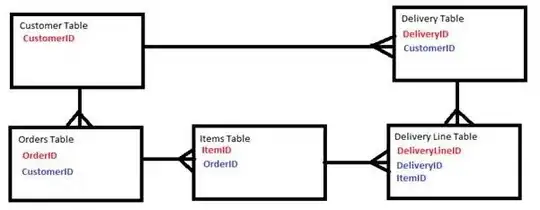
in case of anyone looking for a way to do it, this is how:
based on this post answer , which worked great for me.
this is what I did, I created two drawables one for On and another for Off :
switch_on.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_enabled="false" android:state_checked="true" android:drawable="@color/colorGray"/>
<item android:drawable="@color/colorPrimary" android:state_checked="true" />
<item android:drawable="@color/colorPrimaryDark" android:state_pressed="true" />
<item android:drawable="@color/transparent" />
</selector>
switch_off.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_enabled="false" android:state_checked="true" android:drawable="@color/colorGray"/>
<item android:drawable="@color/gray_light" android:state_checked="true" />
<item android:drawable="@color/black_overlay" android:state_pressed="true" />
<item android:drawable="@color/transparent" />
</selector>
Next , created a drawable for the outline of the switch.
outline.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<corners android:radius="2dp" />
<solid android:color="#80ffffff" />
<stroke
android:width="1dp"
android:color="@color/gray_light" />
</shape>
one thing that I added is a drawable for the text color, because the color of the text changes depending on whether it's checked or not, this is it :
switch_text.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true" android:color="@color/colorWhite"/>
<item android:state_checked="true" android:color="@color/colorWhite"/>
<item android:color="@color/gray_light"/>
</selector>
and finally, created RadioGroup
in my layout this way:
<RadioGroup
android:id="@+id/toggle"
android:layout_width="wrap_content"
android:layout_height="50dp"
android:background="@drawable/outline"
android:checkedButton="@+id/off"
android:orientation="horizontal">
<RadioButton
android:id="@+id/off"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginBottom="1dp"
android:layout_marginStart="1dp"
android:layout_marginTop="1dp"
android:layout_weight="1"
android:background="@drawable/switch_off"
android:button="@null"
android:gravity="center"
android:padding="@dimen/fab_margin"
android:text="@string/off"
android:textColor="@drawable/switch_text" />
<RadioButton
android:id="@+id/on"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_marginBottom="1dp"
android:layout_marginEnd="1dp"
android:layout_marginTop="1dp"
android:layout_weight="1"
android:background="@drawable/switch_on"
android:button="@null"
android:gravity="center"
android:padding="@dimen/fab_margin"
android:text="@string/on"
android:textColor="@drawable/switch_text" />
</RadioGroup>
Notice the usage of each drawable in the right place:
android:background="@drawable/outline"
for the RadioGroup
android:background="@drawable/switch_off"
for the first RadioButton
android:background="@drawable/switch_on"
for the second RadioButton
android:textColor="@drawable/switch_text"
for both Radio Buttons
And that's all.