Solution:
You can do this for your entire application without having to make all your activities extend a common base class.
The trick is first to make sure you include an Application
subclass in your project. In its onCreate()
, called when your app first starts up, you register an ActivityLifecycleCallbacks
object (API level 14+) to receive notifications of activity lifecycle events.
This gives you the opportunity to execute your own code whenever any activity in your app is started (or stopped, or resumed, or whatever). At this point you can call setRequestedOrientation()
on the newly created activity.
class MyApp extends Application {
@Override
public void onCreate() {
super.onCreate();
// register to be informed of activities starting up
registerActivityLifecycleCallbacks(new ActivityLifecycleCallbacks() {
@Override
public void onActivityCreated(Activity activity,
Bundle savedInstanceState) {
// new activity created; force its orientation to portrait
activity.setRequestedOrientation(
ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
}
....
});
}
}
Any doubts, Please leave a comment.
Note: Add in manifest's <application>
tag: android:name=".MyApp"
Hope it helps.
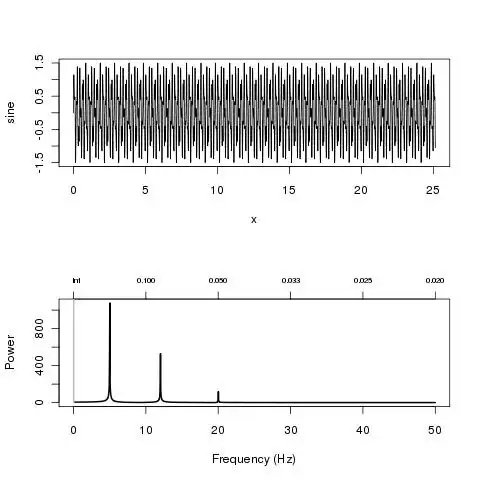