Here is one option. You can create a new GridSpec for each number of subplots you want to show and set the position of the axes according to that gridspec.
import matplotlib.pyplot as plt
from matplotlib.gridspec import GridSpec
from matplotlib.widgets import Button
class VariableGrid():
def __init__(self,fig):
self.fig = fig
self.axes = []
self.gs = None
self.n = 0
def update(self):
if self.n > 0:
for i,ax in zip(range(self.n), self.axes):
ax.set_position(self.gs[i-1].get_position(self.fig))
ax.set_visible(True)
for j in range(len(self.axes),self.n,-1 ):
print(self.n, j)
self.axes[j-1].set_visible(False)
else:
for ax in self.axes:
ax.set_visible(False)
self.fig.canvas.draw_idle()
def add(self, evt=None):
self.n += 1
self.gs= GridSpec(self.n,1)
if self.n > len(self.axes):
ax = fig.add_subplot(self.gs[self.n-1])
self.axes.append(ax)
self.update()
def sub(self, evt=None):
self.n = max(self.n-1,0)
if self.n > 0:
self.gs= GridSpec(self.n,1)
self.update()
fig = plt.figure()
btn_ax1 = fig.add_axes([.8,.02,.05,.05])
btn_ax2 = fig.add_axes([.855,.02,.05,.05])
button_add =Button(btn_ax1, "+")
button_sub =Button(btn_ax2, "-")
grid = VariableGrid(fig)
button_add.on_clicked(grid.add)
button_sub.on_clicked(grid.sub)
plt.show()
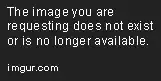