If you use something like an IntVar()
to track the value you can see that it is auto updated with a function that will check the current value.
If you want the value to be displayed and returned as a float you can use DoubleVar()
and then also set resolution=0.01
as an argument in the Scale widget.
import tkinter as tk
class Example(tk.Tk):
def __init__(self):
super().__init__()
self.int_var = tk.IntVar()
self.scale = tk.Scale(self, from_=1, to=100, variable=self.int_var)
self.scale.pack()
tk.Button(self, text="Check Scale", command=self.check_scale).pack()
def check_scale(self):
print(self.int_var.get())
if __name__ == "__main__":
Example().mainloop()
Results:
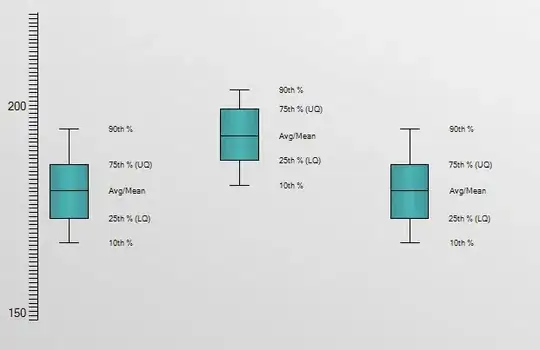
For an example using the DoubleVar()
you can do this:
import tkinter as tk
class Example(tk.Tk):
def __init__(self):
super().__init__()
self.dou_var = tk.DoubleVar()
self.scale = tk.Scale(self, from_=1, to=100, resolution=0.01, variable=self.dou_var)
self.scale.pack()
tk.Button(self, text="Check Scale", command=self.check_scale).pack()
def check_scale(self):
print(self.dou_var.get())
if __name__ == "__main__":
Example().mainloop()
Results:
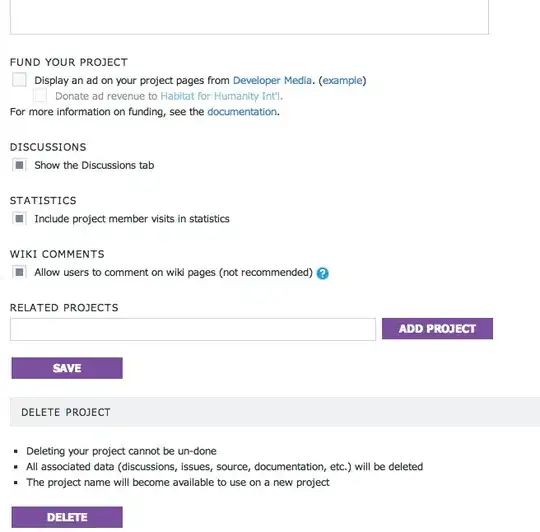