You'll probably have to play a bit around with width and height attributes, but this should get you started. Basically, you're just converting the image/links to html, then using the df.to_html to display those tags. Note, it won't show if you're working in an IDE like PyCharm, Spyder, but as you can see below with my output, works fine through jupyter notebooks
import pandas as pd
from IPython.core.display import display,HTML
df = pd.DataFrame([['A231', 'Book', 5, 3, 150],
['M441', 'Magic Staff', 10, 7, 200]],
columns = ['Code', 'Name', 'Price', 'Net', 'Sales'])
# your images
images1 = ['https://vignette.wikia.nocookie.net/2007scape/images/7/7a/Mage%27s_book_detail.png/revision/latest?cb=20180310083825',
'https://i.pinimg.com/originals/d9/5c/9b/d95c9ba809aa9dd4cb519a225af40f2b.png']
images2 = ['https://static3.srcdn.com/wordpress/wp-content/uploads/2020/07/Quidditch.jpg?q=50&fit=crop&w=960&h=500&dpr=1.5',
'https://specials-images.forbesimg.com/imageserve/5e160edc9318b800069388e8/960x0.jpg?fit=scale']
df['imageUrls'] = images1
df['otherImageUrls'] = images2
# convert your links to html tags
def path_to_image_html(path):
return '<img src="'+ path + '" width="60" >'
pd.set_option('display.max_colwidth', None)
image_cols = ['imageUrls', 'otherImageUrls'] #<- define which columns will be used to convert to html
# Create the dictionariy to be passed as formatters
format_dict = {}
for image_col in image_cols:
format_dict[image_col] = path_to_image_html
display(HTML(df.to_html(escape=False ,formatters=format_dict)))
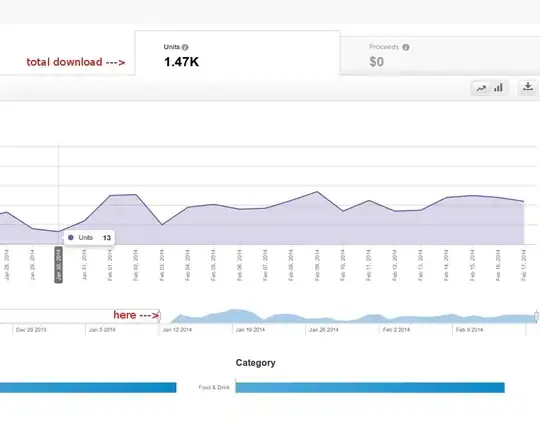
Then you have some options of what to do there to go to pdf.
You could save as html
df.to_html('test_html.html', escape=False, formatters=format_dict)
then simply use and html to pdf converter here, or use a library such as pdfkit or WeasyPrint. I'm not entirely familiar with those (I only used one of them once a long time ago), but here's a good link
Good luck.