This looks like a very wrong idea.
Because
you'll have to visit all the triangles anyway (at least all the visible ones, see below);
connectivity alone is not sufficient; a geometric test is required anyway to take the position of the viewing point into account.
Whatever you do, the complexity has to be linear in the number of visible faces, which is also linear in the total number of faces, and you will trade a straightforward loop on the faces for a complicated graph traversal plus the same amount of geometric work. My bet is a slow down by a factor five or so.
If your surface is convex, the front and rear faces are separated by a continuous contour line. Starting from a visible face, you can "fill" the contiguous faces until you reach that separating line, using a seed fill procedure in the graph. This way, you needn't traverse the rear faces (or just those in contact with the separating line).
This is in fact the method that you describe. Seed filling is essentially a recursive procedure. You can simulate recursion with a local stack, but that will provide no speedup (merely avoid a stack overflow).
There is no way to avoid recursion for a good reason: when you fill the domain from face to face keeping connexity, you can very well end-up with the unfilled area being disconnected. No traversal order can avoid that, and it means that at some stage several regions will have to be filled independently and you need to keep a trace of them. That requires some storage and a backtracking process.
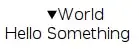
Note also, that on a convex body, there are about as many front faces as rear ones, and the benefit of skipping half of them is largely compensated by the cost of graph traversal and filling.
And if the surface is concave, you may need several starting faces (which I doubt you can efficiently find), as there can be several contours.
Final remark: in the case of a convex surface, you can maybe find the separating contour by starting from the initial face, marching in a direction opposite to the viewing point until you reach some border face and following the contour by tracking the separating edges. The number of faces to visit will be roughly proportional to the number of faces seen during the march to the first contour face, plus the contour length.
For a mesh of F faces, the workload will be of order O(√F). But this still doesn't tell you which are the visible faces.