The idea is to iterate through the items and verify the conditions:
from PyQt4 import QtCore, QtGui
import random
class Widget(QtGui.QWidget):
def __init__(self):
super(Widget, self).__init__()
self.tree = QtGui.QTreeWidget(columnCount=3)
self.tree.setHeaderLabels(["column {}".format(i) for i in range(3)])
button = QtGui.QPushButton("Press Me",
clicked=self.on_clicked)
lay = QtGui.QVBoxLayout(self)
lay.addWidget(self.tree)
lay.addWidget(button)
self.create_data()
self.resize(640, 480)
def create_data(self):
for i in range(4):
it = QtGui.QTreeWidgetItem(["file{}".format(i)])
self.tree.addTopLevelItem(it)
for j in range(random.randint(2, 5)):
vals = ["+", "found item-{}-{}".format(i, j), "new item-{}-{}".format(i, j)]
child_it = QtGui.QTreeWidgetItem(vals)
child_it.setCheckState(2, random.choice([QtCore.Qt.Checked, QtCore.Qt.Unchecked]))
it.addChild(child_it)
self.tree.expandAll()
@QtCore.pyqtSlot()
def on_clicked(self):
d = dict()
for i in range(self.tree.topLevelItemCount()):
top_item = self.tree.topLevelItem(i)
v = []
for j in range(top_item.childCount()):
child_item = top_item.child(j)
if child_item.checkState(2) == QtCore.Qt.Checked:
v.append(tuple(child_item.text(i) for i in (1, 2)))
d[top_item.text(0)] = v
print(d)
if __name__ == '__main__':
import sys
app = QtGui.QApplication(sys.argv)
w = Widget()
w.show()
sys.exit(app.exec_())
Output:
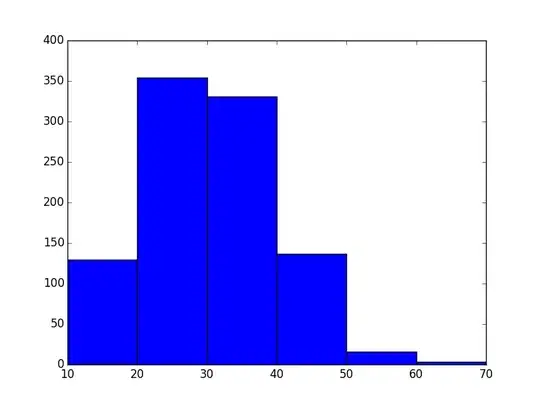
{'file0': [('found item-0-2', 'new item-0-2'), ('found item-0-3', 'new item-0-3')], 'file1': [('found item-1-0', 'new item-1-0'), ('found item-1-1', 'new item-1-1'), ('found item-1-2', 'new item-1-2')], 'file2': [('found item-2-0', 'new item-2-0'), ('found item-2-1', 'new item-2-1'), ('found item-2-3', 'new item-2-3')], 'file3': [('found item-3-0', 'new item-3-0'), ('found item-3-1', 'new item-3-1')]}