I generated a truth table with 256 terms and reduced it to 47 terms using the Espresso minimizer:
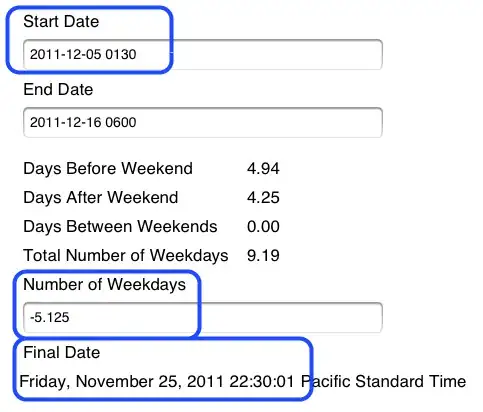
Then, I converted the compressed table into a multi-level circuit (too big to be displayed here as image). For this, I used Logic Friday 1.
It might be possible to derive a simpler circuit by mapping my C# routine into gates:
private static int countConsecutiveBits(int i)
{
int bMax = 0;
int b = 0;
for (int j = 0; j < 8; j++)
{
if (((i >> j) & 1) == 1)
{
b++;
if (bMax < b)
{
bMax = b;
}
}
else
{
b = 0;
}
}
return bMax;
}
This would involve adders and comparators as indicated in your question.
The following circuit - hand crafted and not verified - is a bit-slice which takes as inputs the so-far maximum bit count, the current bit count and the respective bit. The outputs are the new maximum bit count and the current bit count. All counts are 4-bit wide. This could be optimized for the first slices, where the count is smaller.
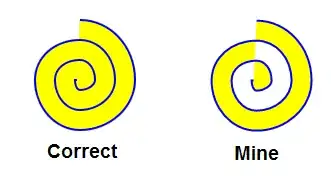