Source => Detection
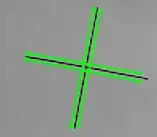
My method is: Gray => Threshold => FindContours (filter by area if necessary)
#!/usr/bin/python3
# 2019/03/01
import cv2
img = cv2.imread("featmap.png")
# Gray => Threshold => FindContours (filter by area if necessary)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blured = cv2.medianBlur(gray, 3)
th, threshed = cv2.threshold(blured, 100, 255, cv2.THRESH_OTSU|cv2.THRESH_BINARY)
cnts = cv2.findContours(threshed, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)[-2]
print("nums: {}".format(len(cnts)))
# draw on the original
cv2.drawContours(img, cnts, -1, (0, 255, 0), 1, cv2.LINE_AA)
cv2.imwrite("dst.png", img)
Be carefully when using findContours
: How to use `cv2.findContours` in different OpenCV versions?