There seems to be two ways of deciding how many cells a grid item spans:
To be more precise there are 3 ways to place items. From the specification:
The contents of the grid container are organized into individual grid items (analogous to flex items), which are then assigned to predefined areas in the grid. They can be explicitly placed using coordinates through the grid-placement properties or implicitly placed into empty areas using auto-placement. §8 Placing Grid Items
So either you consider area, coordinate or you leave the job to the browser for the auto placement. Basically you can use only one way.
Note that grid-area
is the shorthand property for the explicit placement which can also be replace by grid-row-start; grid-column-star; grid-row-end; grid-column-end;
Here is a simple example to illustrate:
.grid {
display:inline-grid;
grid-template-areas:
"a b"
"c d";
grid-gap:20px;
border:1px solid;
}
span {
width:50px;
height:50px;
background:red;
}
.one > span {
grid-area:a;
grid-row-start:1;
grid-row-end:3;
grid-column-start:1;
grid-column-end:3;
}
.two > span {
grid-row-start:1;
grid-row-end:3;
grid-column-start:1;
grid-column-end:3;
grid-area:a;
}
<div class="grid one">
<span></span>
</div>
<div class="grid two">
<span></span>
</div>
You can clearly see that we have a different result because of the order. This is logical since we are overriding properties. There isn't any order but only one configuration will be considered for your item.
You can inspect the second element and you will have the computed value like this:
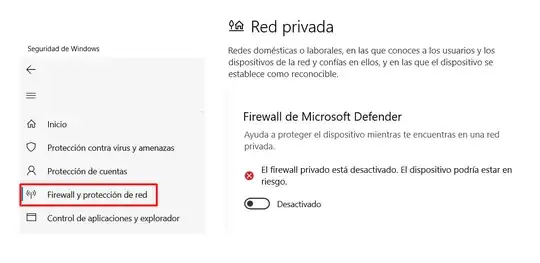
You will not find a property called grid-area
like you won't find a property called background
, margin
, border
etc because all will get replaced by the longhand notation.
Considering the size, you should make the difference between the size of the tracks and the size of the items. In the previous example we didn't define any explicit size so the item width/height will also define the size of the track.
You can explicitely set the track sizes using different properties like grid-template-columns
grid-template-rows
and you will notice that grid item size will not always follow the size of the tracks and we may have overflow:
.grid {
display:inline-grid;
grid-template-columns:150px 150px;
grid-template-areas:
"a b"
"c d";
grid-gap:20px;
border:1px solid;
}
span {
width:50px;
height:50px;
background:red;
grid-area:a;
}
.one > span {
width:400px;
}
.two > span {
width:100%;
}
.three > span {
width:200%;
}
<div class="grid one">
<span></span>
</div>
<div class="grid two">
<span></span>
</div>
<div class="grid three">
<span></span>
</div>
We have defined the track size to be 150px
and if the item with is bigger we simply have an overflow. You will also notice how percetange will behave since the track will be the containing block of the grid item and not the grid container.
Using dev tools you can clearly see the tracks:
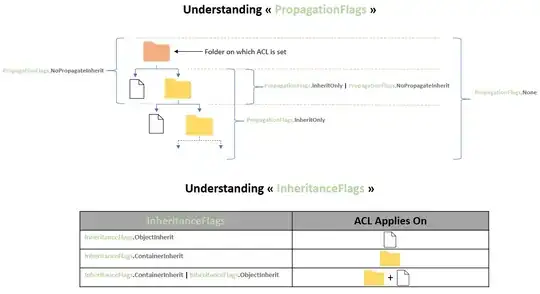
If for example you consider 1fr
unit or auto
then the width of the element will be used to define the sizes:
.grid {
display:inline-grid;
grid-template-columns:1fr 150px;
grid-template-areas:
"a b"
"c d";
grid-gap:20px;
border:1px solid;
}
span {
width:50px;
height:50px;
background:red;
grid-area:a;
}
.one > span {
width:400px;
}
<div class="grid one">
<span></span>
</div>
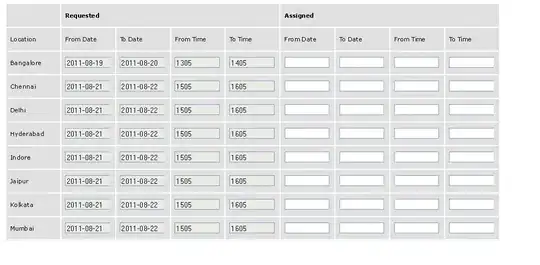
So we can identify 4 cases 1:
- item size not defined and track size not defined: The content of each item will be used to define the item and the track size.
- item size not defined and track size defined: The item will be stretched to fill the track size (we may have overflow depending on the content of the item).
- item size defined and track size defined: There is no size relation between both, we simply place the item inside the track. We can either have overflow or empty space inside the track.
- item size defined and track size not defined: The item size will dictate the track size. Depending on the cases, it will not define it but it will be considered in the caclulation. (ex here: https://stackoverflow.com/a/54639430/8620333)
1: this is very simplifed to make think more clear. The sizing algorithm is more complex.