In the approach below, we find the cumulative area of overlap and then find the x value at which this cumulative area is half of the total overlap area.
For illustration, I've added additional data columns to mark all the steps, but that's not necessary if you just want to find the location of the dividing line directly.
# overlap
mydf$overlap = apply(mydf[,c("y1","y2")], 1, min)
# area of overlap
mydf$overlap.area = cumsum(mydf$overlap * median(diff(mydf$x)))
# Find x that divides overlap area in half
# Method 1: Directly from the data. Could be inaccurate if x values are
# not sufficiently close together.
x0a = mydf$x[which.min(abs(mydf$overlap.area - 0.5*max(mydf$overlap.area)))]
# Method 2: Use uniroot function to find x value where cumulative overlap
# area is 50% of total overlap area. More accurate.
# First generate an interpolation function for cumulative area.
# Subtract half the cumulative area so that function will cross
# zero at the halfway point
f = approxfun(mydf$x, mydf$overlap.area - 0.5*max(mydf$overlap.area))
# Find x value at which interpolation function crosses zero
x0b = uniroot(f, range(mydf$x))$root
p0 = ggplot(mydf, aes(x = x)) +
geom_line(aes(y = y1), colour = 'blue') +
geom_line(aes(y = y2), colour = 'red') +
geom_area(aes(y = pmin(y1, y2)), fill = 'gray60') +
geom_line(aes(y=overlap), colour="purple") +
geom_line(aes(y=overlap.area), colour="green") +
geom_vline(xintercept=c(x0a,x0b), color=c("orange","darkgreen"),
linetype=c("solid", "dashed")) +
theme_classic()
p0
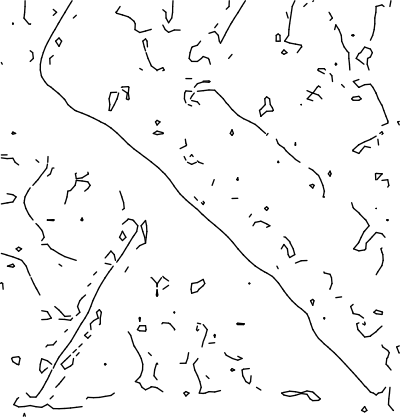