iOS 15
from iOS 15 and with the SFSymbols 3, you can apply different layers of colors to a single symbol with the foreground style modifier:
Image(systemName: "person.circle")
.resizable()
.foregroundStyle(.red, .blue)
.frame(width: 200, height: 200, alignment: .center)
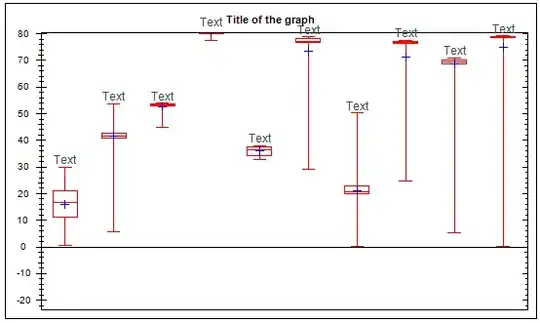
iOS 13 and 14
You can use a ZStack
with different parts of the icon and apply different modifiers to each layer like:
/// I've used `GeometryReader ` for setting the size of elements dependent on each other
GeometryReader { proxy in
ZStack {
Image(systemName: "circle")
.resizable()
.foregroundColor(.blue)
Image(systemName: "person.fill")
.resizable()
.foregroundColor(.red)
.frame(
width: proxy.size.width * 0.55,
height: proxy.size.width * 0.55,
alignment: .center
)
}
}.frame(width: 200, height: 200, alignment: .center)
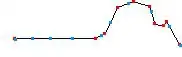
Note that the old and new methods look slightly different but feel the same. (take a closer look at the roundness of the head)