The best practice is to use IAM roles for AWS APIs. Because you don't need to worry about managing AWS credentials. Use IAM roles to manage AWS resources internally, and use IAM users to manage AWS resources externally. So here I'm not going to compare the differences furthermore, I'll attach a few links for your reference.
No need to specify AWS credentials with boto3 whether you use IAM roles or IAM users. Here are the workarounds for IAM roles and IAM users.
You can find the below configurations under the Job configuration section.
Let's assume you have an IAM roles that has permission for relevant AWS resources. Just select it from the drop-down menu.
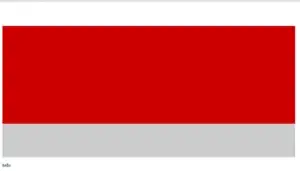
Let's assume you have an IAM users account that has permission for relevant AWS resources. Just enter credential details where you get them when you create an IAM users account to set them as environment variables. In this way, you don't need to hardcode the AWS credentials.
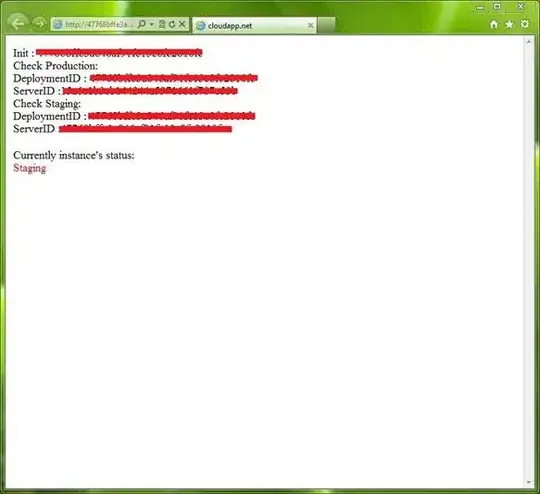
You can choose either IAM roles or IAM users based on your requirement. But whatever the thing you choose, you don't need to specifically mention the aws_access_key_id
and aws_secret_access_key
. Sometimes you have to mention region_name
, this is affected both ways. Because I faced this kind of issue a few years back.
Anyway if you didn't specifically mention the credentials when you create the boto3 client object, the default value None
will be assigned. If the None
is assigned it will check the environment variables, otherwise, will give an error. That's why mentioned, "you don't need to specifically mention the aws_access_key_id
and aws_secret_access_key
".
KMS = boto3.client('kms') # better if you can mention "region_name" here
Hope everything is clear, let me know if you are faced with any difficulties.
References:-