I made a simple program to select all the Hello
s in the Text:
from tkinter import *
def find_nth(haystack, needle, n): #Function to find the index of nth substring in a string
start = haystack.find(needle)
while start >= 0 and n > 1:
start = haystack.find(needle, start+len(needle))
n -= 1
return start
def find():
word = "H" #Targetted Word
text, line = tx.get("1.0",END), 0 #text getting text of the widget
text = text.split("\n") #splitting and getting list on the newlines
for x, i in enumerate(text): #Looping through that list
if word in i: #if targetted word is in the xth string i of the list
for e in range(0, i.count(word)):
index = find_nth(i, word, e+1) #Getting the index of the word
start = float(str(x+1)+"."+str(index)) #Making the indices for tkinter
end = float(str(x+1)+"."+str(index+len(word))) #Making the indices for tkinter
tx.focus() #Focusing on the Text widget to make the selection visible
tx.tag_add("sel", start, end) #selecting from index start till index end
root = Tk()
tx = Text(root)
tx.insert(END, "World Hello World\nHello World Hello Hello\nHello Hello")
bu = Button(root, text = "Find Hello", command = find)
tx.pack()
bu.pack()
root.mainloop()
Output:
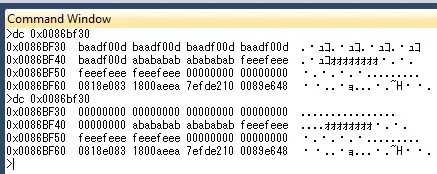
I used this answer for help.