A simple method is to extract the ROI using Numpy slicing, pixelate, then paste it back into the original image. I will be using the pixelation technique found in how to pixelate image using OpenCV in Python?. Here's a simple example:
Input image and ROI to be extracted
Extracted ROI

Pixelated ROI
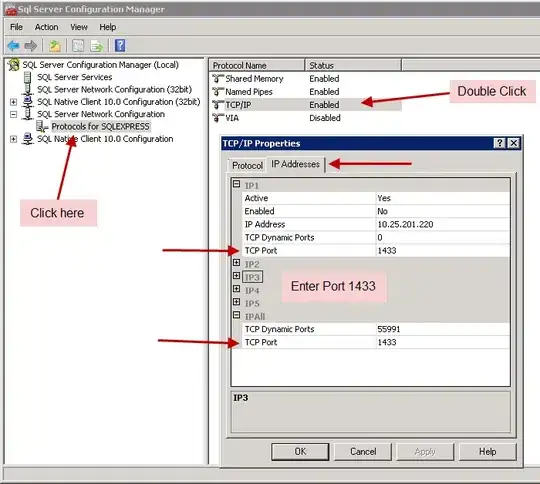
Result
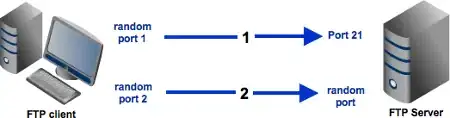
Code
import cv2
def pixelate(image):
# Get input size
height, width, _ = image.shape
# Desired "pixelated" size
h, w = (16, 16)
# Resize image to "pixelated" size
temp = cv2.resize(image, (w, h), interpolation=cv2.INTER_LINEAR)
# Initialize output image
return cv2.resize(temp, (width, height), interpolation=cv2.INTER_NEAREST)
# Load image
image = cv2.imread('1.png')
# ROI bounding box coordinates
x,y,w,h = 122,98,283,240
# Extract ROI
ROI = image[y:y+h, x:x+w]
# Pixelate ROI
pixelated_ROI = pixelate(ROI)
# Paste pixelated ROI back into original image
image[y:y+h, x:x+w] = pixelated_ROI
cv2.imshow('pixelated_ROI', pixelated_ROI)
cv2.imshow('image', image)
cv2.waitKey()
Note: The ROI bounding box coordinates were found by using the script in how to get ROI Bounding Box Coordinates without Guess & Check. For your case, I will assume that you already have the x,y,w,h
bounding box coordinates obtained by cv2.boundingRect
.