Since you are using ttk
package, you have to use a Style
to apply any modifications to widgets used from that package.
To dynamically change them, such as with a button press, pass a reference to the widget to a function to do whatever you like. I personally decided to also pass a reference to the Style
object so it doesn't need to be recreated with each function call. Modify this example code to fit your needs.
import tkinter as tk
# Using cycle for demonstration purposes
from itertools import cycle
from tkinter import ttk
class TKTEST:
def __init__(self) -> None:
self.tkapp()
def tkapp(self) -> None:
root = tk.Tk()
root.title("Test Dynamic Color Change")
root.geometry("300x300")
# Create style object before button
style = ttk.Style()
# Create a collection of colors to cycle through
colors = cycle(["#e9c46a","#e76f51","#264653","#2a9d8f","#e85d04","#a2d2ff","#06d6a0","#4d908e"])
# Using pack() to stretch out the frame to the size of the window
mainframe = ttk.Frame(master=root, relief="groove")
mainframe.pack(fill="both", expand=True)
# Use place() to center the button horizontally and vertically in the frame
# Use a lambda function to be able to pass arguments to the called function
button = ttk.Button(master=mainframe, text="Change Colors", command=lambda: self.change_frame_color(mainframe, style, colors))
button.place(relx=0.5, rely=0.5, anchor="center")
root.mainloop()
# Using type hinting with method arguments
def change_frame_color(self, objFrame: ttk.Frame, style: ttk.Style, colors: cycle) -> None:
# First, apply a style to frame since we're using ttk package
objFrame.configure(style="Mainframe.TFrame")
# Second, configure the style with a background color
style.configure("Mainframe.TFrame", background=next(colors))
tktest = TKTEST()
Changing the frame's color with each button press:
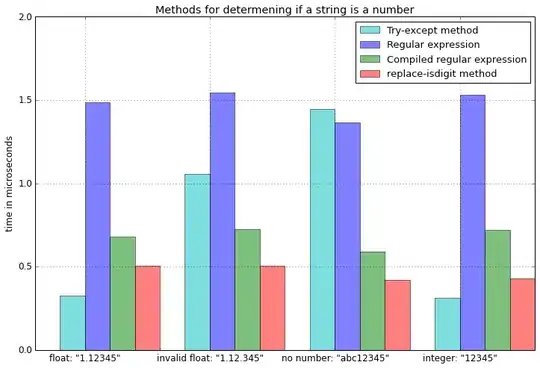