Indentation
As said in the comments by jasonharper and Daniel Huckson, the indentation can be changed with
style.configure('Treeview', indent=100)
Indicator image
The indicator image can be changed by creating a custom theme element and using it in replacement of the standard indicator in the Treeview.Item
layout.
The key point here is to know the names of the states of an opened item ('user1') and of an item without children ('user2'), closed being the default state. Therefore the open indicator needs to be mapped with items in the state ('user1', '!user2')
and the empty image to the items in state ('user2', )
.
I used PIL to create dummy images for the indicator, but one can directly load custom images instead.
from PIL import Image, ImageTk, ImageDraw
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
style = ttk.Style(root)
# custom indicator images
im_open = Image.new('RGBA', (15, 15), '#00000000')
im_empty = Image.new('RGBA', (15, 15), '#00000000')
draw = ImageDraw.Draw(im_open)
draw.polygon([(0, 4), (14, 4), (7, 11)], fill='yellow', outline='black')
im_close= im_open.rotate(90)
img_open = ImageTk.PhotoImage(im_open, name='img_open', master=root)
img_close = ImageTk.PhotoImage(im_close, name='img_close', master=root)
img_empty = ImageTk.PhotoImage(im_empty, name='img_empty', master=root)
# custom indicator
style.element_create('Treeitem.myindicator',
'image', 'img_close', ('user1', '!user2', 'img_open'), ('user2', 'img_empty'),
sticky='w', width=15)
# replace Treeitem.indicator by custom one
style.layout('Treeview.Item',
[('Treeitem.padding',
{'sticky': 'nswe',
'children': [('Treeitem.myindicator', {'side': 'left', 'sticky': ''}),
('Treeitem.image', {'side': 'left', 'sticky': ''}),
('Treeitem.focus',
{'side': 'left',
'sticky': '',
'children': [('Treeitem.text', {'side': 'left', 'sticky': ''})]})]})]
)
tree = ttk.Treeview(root)
tree.pack()
tree.insert('', 'end', text='item 1', open=True)
tree.insert('', 'end', text='item 2')
tree.insert('I001', 'end', text='item 11', open=False)
tree.insert('I001', 'end', text='item 12', open=False)
tree.insert('I004', 'end', text='item 121', open=False)
root.mainloop()
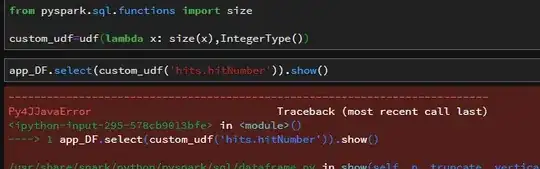