Here is some code which shows how this can be achieved:
Assume the following starting point:
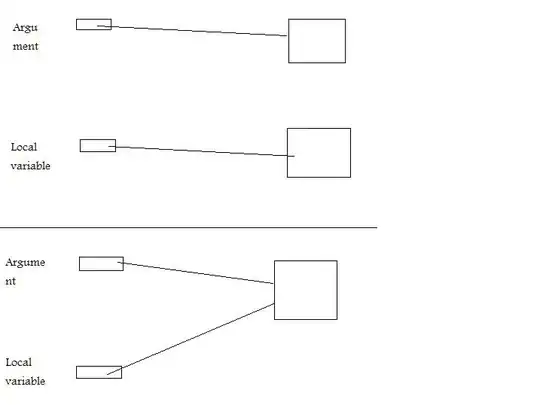
And the following "extra" row of data in a JavaScript array variable:
var extraData = [
"David",
"Software Engineer 2",
"Tokyo",
25,
"04/25/2011",
320800
];
Then when the data is exported to Excel, the table looks like this:
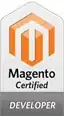
The DataTables code:
<script type="text/javascript">
$(document).ready(function() {
var extraData = [
"David",
"Software Engineer",
"Tokyo",
29,
"04/25/2011",
320800
];
var table = $('#example').dataTable({
dom: 'Bfrtip',
buttons: [
{
extend: 'excelHtml5',
title: '', // no title row
text: 'Excel',
customize: function ( xlsx ){
var sheet = xlsx.xl.worksheets['sheet1.xml'];
insertRowAfter( 6, sheet );
populateRow( 7, extraData, sheet );
}
}
]
});
// Insert a new row after the rowNum row. The new row is
// cloned from the rowNum row.
function insertRowAfter(rowNum, sheet) {
var $src_row = $( 'row[r=' + rowNum + ']', sheet );
var newRowID = parseInt( $src_row.attr( 'r' ) ) + 1;
var $new_row = $src_row.clone().attr( 'r', newRowID );
// fix the cell references in the new row:
$new_row.children( 'c' ).each(function( index ) {
var newCellID = $( this ).attr( 'r' ).match(/[A-Z]+/) + newRowID
$( this ).attr( 'r', newCellID );
});
// Increment the row and cell references in all rows below
// where the new row will be inserted:
$src_row.nextAll('row').each(function( index ) {
var nextRowID = parseInt( $( this ).attr( 'r' )) + 1;
$( this ).attr( 'r', nextRowID );
$( this ).children( 'c' ).each(function( index ) {
var nextCellID = $( this ).attr( 'r' ).match(/[A-Z]+/) + nextRowID
$( this ).attr( 'r', nextCellID );
});
});
// Insert the new row:
$src_row.after($new_row);
}
function populateRow( row, data, sheet ) {
// Assumes data starts in column A, with no gaps. Assumes
// no more than 26 columns (A through Z).
data.forEach(function (item, index) {
var col = String.fromCharCode(65 + index); // 65 = ascii 'A'
if ( typeof item === 'string' ) {
$('c[r=' + col + row + '] t', sheet).text( item );
} else if ( typeof item === 'number' ) {
$('c[r=' + col + row + '] > v', sheet).html( item );
}
});
}
});
</script>
Points to Note:
1) I changed your JS {...}
object for the "extra row" data to an array [...]
. You can alter the code and change it back if you prefer - but for my demo, I wanted to keep it simple.
2) The data in the "extra row" array has to be formatted in a specific way - by which I mean: For numbers, make sure the data is not in quotation marks. And, for example, the dollar amount is not formatted with any commas or dollar signs. Let Excel do the formatting for you.
3) This code is somewhat basic, and Excel sheets are fairly complex. For the specific scenario in your question, this works. But it's quite possible that the code will need to be adjusted to handle other types of data - or empty/blank values, and so on. Just to warn you.
I would say, do what you can to avoid needing to insert new rows like this. Or use a library which is probably better equipped to handle this.
Edit
Assuming we start with an object {...}
instead of an array [...]
:
var dataObject = {
Name: "David",
Position: "Software Engineer",
Office: "Tokyo",
Age: 29,
"Start date": "2011/04/25",
Salary: 320800
};
There are various ways to handle this.
Here is one way, where we convert the data to an array, before using it:
var extraData = [];
for(var o in dataObject) {
extraData.push(dataObject[o]);
}
Now we can use extraData
in the same way as shown above.