Here's why your answer wasn't working:
See this about the yticklabels
argument in the documentation:
If list-like, plot these alternate labels as the xticklabels.
So basically when you only pass a few tick labels, it is just setting those names as the tick labels, without knowledge of the tick positions. One way to get around this is to do the following, adding sample_labels
which makes a label for all ticks, but sets non-interesting ones to None
. You then follow this answer to rotate the ticks):
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
iris = sns.load_dataset('iris')
samples = ['sample_'+str(x) for x in list(iris.index)]
iris.insert(0,'Sample_ID',samples)
samples_of_interest = ['sample_41','sample_34','sample_114','sample_55']
sample_labels = [i if i in samples_of_interest else None
for i in iris['Sample_ID'] ]
cm=sns.clustermap(iris.iloc[:,1:-1], yticklabels=sample_labels)
plt.setp(cm.ax_heatmap.yaxis.get_majorticklabels(), rotation=0)
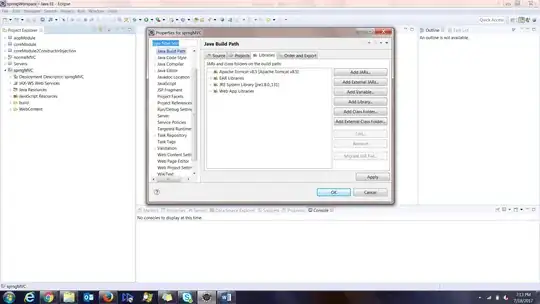
But this is still not ideal b/c there are ticks for all the positions I'm sure there is a way to edit this but instead..
Here's a method I like more:
Get the new order of the samples from the clustergrid
(object returned by clustermap
, then manually set the y-tick labels and positions (with credit to this post):
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
iris = sns.load_dataset('iris')
samples_of_interest = [41, 34, 114, 55]
sample_names = ['Sample ' + str(i) for i in samples_of_interest]
cm=sns.clustermap(iris.iloc[:,:-1]) #note the loc has changed!
reorder = cm.dendrogram_row.reordered_ind
new_positions = [reorder.index(i) for i in samples_of_interest]
plt.setp(cm.ax_heatmap.yaxis.set_ticks(new_positions))
plt.setp(cm.ax_heatmap.yaxis.set_ticklabels(sample_names))

Oddly the cm.ax_heatmap.yaxis.set...
commands print out the get
versions (it seems), but this doesn't affect outcome