You are welcome to check my answer to a similar question here: https://stackoverflow.com/questions/12096152/plotting-symbols-fails-in-pdf/63214207?r=SearchResults&s=2|0.0000#63214207
But here is the solution for your problem.
#--- A function to install missing packages and load them all
myfxLoadPackages = function (PACKAGES) {
lapply(PACKAGES, FUN = function(x) {
if (suppressWarnings(!require(x, character.only = TRUE))) {
install.packages(x, dependencies = TRUE, repos = "https://cran.rstudio.com/")
}
})
lapply(PACKAGES, FUN = function(x) library(x, character.only = TRUE))
}
packages = c("ggplot2","gridExtra","grid","png")
myfxLoadPackages(packages)
#--- The trick to get unicode characters being printed on pdf files:
#--- 1. Create a temporary file, say "temp.png"
#--- 2. Create the pdf file using pdf() or cairo_pdf(), say "UnicodeToPDF.pdf"
#--- 3. Combine the use of grid.arrange (from gridExtra), rasterGrob (from grid), and readPNG (from png) to insert the
# temp.png file into the UnicodeToPDF.pdf file
test.plot = ggplot() +
geom_point(data = data.frame(x=1, y=1), aes(x,y), shape = "\u2191", size=3.5) +
geom_point(data = data.frame(x=2, y=2), aes(x,y), shape = "\u2020", size=3.5) +
geom_point(data = data.frame(x=1.2, y=1.2), aes(x,y), shape = -10122, size=3.5, color="#FF7F00") +
geom_point(data = data.frame(x=1.4, y=1.4), aes(x,y), shape = -129322, size=3.5, color="#FB9A99") +
geom_point(data = data.frame(x=1.7, y=1.7), aes(x,y), shape = -128515, size=5, color="#1F78B4")
ggsave("temp.png", plot = test.plot, width = 80, height = 80, units = "mm")
#--- Refer to http://xahlee.info/comp/unicode_index.html to see more unicode character integers
pdf("UnicodeToPDF.pdf")
grid.arrange(
rasterGrob(
readPNG(
"temp.png",
native=F
)
)
)
dev.off()
file.remove("temp.png")
The following image has been added to follow up on Konrad Rudolph's comments.
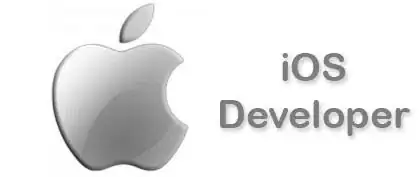