CSS solution
Based on changing the parameters of the stroke-dasharray attribute
To set the desired attribute values in accordance with the opening angle of the sector, you need to calculate the total circumference at the selected radius.
Let's say the radius is 50px
let radius = 50;
let circumference = radius * 2 * Math.PI;
console.log(circumference );
Full circumference ~= 314px
For example, to draw a segment equal to a quarter of a circle:
calculate the length of the dash: 314 * 0.25 = 78.5px
gap length: 314 * 0.75 = 235.5px
Formula for stroke-dasharray: stroke-dasharray="78.5, 235.5"
Similarly, stroke-dasharray parameters are set for other corners of sectors.
circle {
fill:#665555;
}
#p15,#p90,#p180,#p270 {
fill:none;
stroke:#9ACD32;
stroke-width:100;
}
#p15 {
stroke-dasharray:15.7,298.3;
}
#p90 {
stroke-dasharray:78.5,235.5;
}
#p180 {
stroke-dasharray:157,157;
}
#p270 {
stroke-dasharray:235.5,78.5;
}
<svg width="200" height="200" style="border:1px solid">
<circle id="bg" r="100" cx="100" cy="100" />
<path id="p15" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50">
</path>
</svg>
<!-- 90deg -->
<svg width="200" height="200" style="border:1px solid">
<circle r="100" cx="100" cy="100" />
<path id="p90" stroke-dasharray="78.5,235.5" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50">
</path>
</svg>
<!-- 180deg -->
<svg width="200" height="200" style="border:1px solid">
<circle r="100" cx="100" cy="100" />
<path id="p180" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50">
</path>
</svg>
<!-- 270deg -->
<svg width="200" height="200" style="border:1px solid">
<circle r="100" cx="100" cy="100" />
<path id="p270" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50">
</path>
</svg>
SVG solution
As in the example CSS solution
uses changing the stroke-dasharray
attributes
<svg width="200" height="200">
<circle id="bg" r="100" cx="100" cy="100" fill="#665555"/>
<path stroke-dasharray="300 14" stroke-dashoffset="300" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" id="p1"
r="50" cx="100" cy="100" stroke="#9ACD32" stroke-width="100" fill="none" >
</path>
</svg>
<svg width="200" height="200">
<circle r="100" cx="100" cy="100" fill="#665555"/>
<path stroke-dasharray="235.5 78.5" stroke-dashoffset="235.5" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" stroke="#9ACD32" stroke-width="100" fill="none" >
</path>
</svg>
<svg width="200" height="200">
<circle id="bg" r="100" cx="100" cy="100" fill="#665555"/>
<path stroke-dasharray="157 157" stroke-dashoffset="157" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" stroke="#9ACD32" stroke-width="100" fill="none" >
</path>
</svg>
<svg width="200" height="200">
<circle id="bg" r="100" cx="100" cy="100" fill="#665555"/>
<path stroke-dasharray="78.5 235.5" stroke-dashoffset="78.5" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" stroke="#9ACD32" stroke-width="100" fill="none" >
</path>
</svg>
An example of interactively changing the angle of a sector
The author's wishes:
For context I will be passing data from an array to determine the
percentage of the pie chart.
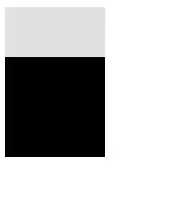
This process is modeled using input
and javascript
:
- Displaying the percentage of filling the chart
let circumference = 50 * 2 * Math.PI,
input = document.querySelector("[type='range']"),
txt = document.querySelector("#txt1");
input.addEventListener("input",()=>{
pieChart();
})
window.addEventListener("load",()=>{
pieChart();
})
function pieChart(){
let val = Number(input.value);
let dash = circumference * val / 100;
let gap = circumference - dash;
p15.style.strokeDasharray = dash + " " + gap
txt.innerHTML = (val + '%');
}
<div><input id="size" step="1" type="range" min="0" max = "100" value="0" /></div>
<svg width="200" height="200" >
<circle id="bg" r="100" cx="100" cy="100" fill="#665555"/>
<path id="p15" stroke-dasharray="15.7,298.3" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" id="p1" fill="none" stroke="#9ACD32" stroke-width="100" >
</path>
<text id="txt1" y="60%" x="50%" text-anchor="middle" font-size="32px" fill="white">0</text>
</svg>
- Chart fill angle output
let circumference = 50 * 2 * Math.PI,
input = document.querySelector("[type='range']"),
txt = document.querySelector("#txt1");
input.addEventListener("input",()=>{
pieChart();
})
window.addEventListener("load",()=>{
pieChart();
})
function pieChart(){
let val = Number(input.value);
let dash = circumference * val / 360;
let gap = circumference - dash;
p15.style.strokeDasharray = dash + " " + gap
txt.innerHTML = (val);
}
<div><input id="size" step="1" type="range" min="0" max = "360" value="0" /></div>
<svg width="200" height="200" >
<circle id="bg" r="100" cx="100" cy="100" fill="#665555"/>
<path id="p15" stroke-dasharray="15.7,298.3" d="M100,50A50,50 0 0 1 100,150A50,50 0 0 1 100,50" id="p1" fill="none" stroke="#9ACD32" stroke-width="100" >
</path>
<text id="txt1" y="60%" x="50%" text-anchor="middle" font-size="32px" fill="white">0</text>
</svg>