Your code currently isn't setting anything, XRSettings.StereoRenderingMode stereoRenderingMode;
is not a reference to the current settings.
All you are doing is creating a new variable stereoRenderingMode
of type XRSettings.StereoRenderingMode
(which is an enum
) and updating that variable its value to XRSettings.StereoRenderingMode.SinglePass
, but after that you don't do anything with this value. it just gets cleaned up at the end of your function.
this is practically the same as doing the following, but instead with the enum StereoRenderingMode
string foo = "Hello";
void Start()
{
foo = "world";
}
I don't think you are able to set the Render mode
at run time (most likely because it needs to be known before building your application). if it would have been possible it would've (most likely) been done by setting the XRSettings.stereoRenderMode
, but that is a read only property, meaning Unity doesn't intend for you to change it at runtime (at least not through that route.). and there is no function like SetStereoRenderMode
either to set it through.
As far as I am aware the only place you can change it is under Project settings > XR Plugin-in management > <Your XR device> > Render Mode
(if you are using the new XR plugin mangement system) 
or else under Project Settings > Player > XR Settings > Stereo Rendering Mode
if you are using the old (deprecated) XR management system. 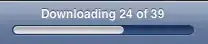
Editing this setting should not be reset after each build/run though, make sure you save your project after changing it to SinglePass
to ensure a reload won't reset it. If it still happens you might want to look as Unity's issue tracker if this is a known bug, and if not report it as a bug as it does sound like unintended behavior. (It doesn't reset back to MultiPass when I try it locally)