Based on the images you've shown, you're doing a few things wrong.
First, for your attempt with a XIB, you've used a UIView
in the xib. It should be a UITableViewCell
.
Second, the prototype layout... most of it looks correct (ish). You've given Class Name Label
leading and trailing constraints and a centerX constraint. The centerX constraint doesn't do anything, because the label will already stretch to the leading and trailing values. Also, your Stack View leading constraint should probably be relative to the trailing edge of the Star Image View, not to the leading of the cell.
Look at the constraints shown here:
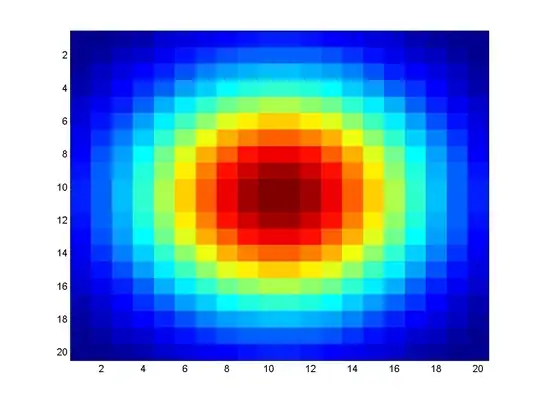
The cell shows as "cell" because that's the Reuse Identifier I've given it, but the Custom Class is set to EducationTableCell
.
The output looks like this:
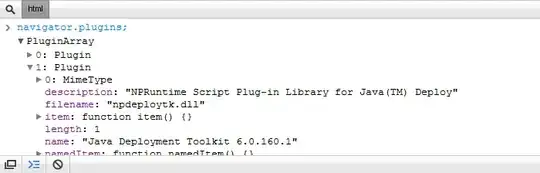
Here is example code to produce that output:
import UIKit
class EducationTableCell: UITableViewCell {
@IBOutlet var className: UILabel!
@IBOutlet var classLocation: UILabel!
@IBOutlet var classDays: UILabel!
@IBOutlet var classTime: UILabel!
@IBOutlet var starImageView: UIImageView!
@IBOutlet var itemImageView: UIImageView!
}
class EducationTableViewController: UITableViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
// MARK: - Table view data source
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 20
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as! EducationTableCell
cell.className.text = "Name \(indexPath.row)"
cell.classLocation.text = "Location \(indexPath.row)"
cell.classDays.text = "Days \(indexPath.row)"
cell.classTime.text = "Time \(indexPath.row)"
return cell
}
}
and here is the source for the Storyboard so you can inspect the layout constraints:
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="17506" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" useSafeAreas="YES" colorMatched="YES" initialViewController="Rco-aF-G6p">
<device id="retina4_7" orientation="portrait" appearance="light"/>
<dependencies>
<deployment identifier="iOS"/>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="17506"/>
<capability name="System colors in document resources" minToolsVersion="11.0"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--Education Table View Controller-->
<scene sceneID="0rL-fH-xZI">
<objects>
<tableViewController id="Rco-aF-G6p" customClass="EducationTableViewController" customModule="PanZoom" customModuleProvider="target" sceneMemberID="viewController">
<tableView key="view" clipsSubviews="YES" contentMode="scaleToFill" alwaysBounceVertical="YES" dataMode="prototypes" style="plain" separatorStyle="default" rowHeight="-1" estimatedRowHeight="-1" sectionHeaderHeight="28" sectionFooterHeight="28" id="Nk0-AL-MEx">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<color key="backgroundColor" systemColor="systemBackgroundColor"/>
<prototypes>
<tableViewCell clipsSubviews="YES" contentMode="scaleToFill" preservesSuperviewLayoutMargins="YES" selectionStyle="default" indentationWidth="10" reuseIdentifier="cell" rowHeight="138" id="SUJ-72-5Po" customClass="EducationTableCell" customModule="PanZoom" customModuleProvider="target">
<rect key="frame" x="0.0" y="28" width="375" height="138"/>
<autoresizingMask key="autoresizingMask"/>
<tableViewCellContentView key="contentView" opaque="NO" clipsSubviews="YES" multipleTouchEnabled="YES" contentMode="center" preservesSuperviewLayoutMargins="YES" insetsLayoutMarginsFromSafeArea="NO" tableViewCell="SUJ-72-5Po" id="G9i-wf-fpQ">
<rect key="frame" x="0.0" y="0.0" width="375" height="138"/>
<autoresizingMask key="autoresizingMask"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="className" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="mVQ-Zs-26O">
<rect key="frame" x="21" y="16" width="333" height="20.5"/>
<color key="backgroundColor" red="0.99953407049999998" green="0.98835557699999999" blue="0.47265523669999998" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<nil key="textColor"/>
<nil key="highlightedColor"/>
</label>
<imageView clipsSubviews="YES" userInteractionEnabled="NO" contentMode="scaleAspectFit" horizontalHuggingPriority="251" verticalHuggingPriority="251" translatesAutoresizingMaskIntoConstraints="NO" id="3yA-G1-vya">
<rect key="frame" x="21" y="56.5" width="50" height="50"/>
<color key="backgroundColor" red="1" green="0.14913141730000001" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstAttribute="width" constant="50" id="TjC-BK-Udr"/>
<constraint firstAttribute="width" secondItem="3yA-G1-vya" secondAttribute="height" multiplier="1:1" id="vWg-SJ-7vR"/>
</constraints>
</imageView>
<imageView clipsSubviews="YES" userInteractionEnabled="NO" contentMode="scaleAspectFit" horizontalHuggingPriority="251" verticalHuggingPriority="251" translatesAutoresizingMaskIntoConstraints="NO" id="cs2-jh-xoS">
<rect key="frame" x="324" y="71.5" width="30" height="30"/>
<color key="backgroundColor" red="1" green="0.14913141730000001" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstAttribute="width" constant="30" id="ndl-Lm-Bz0"/>
<constraint firstAttribute="width" secondItem="cs2-jh-xoS" secondAttribute="height" multiplier="1:1" id="oHy-eo-dLd"/>
</constraints>
</imageView>
<stackView opaque="NO" contentMode="scaleToFill" axis="vertical" spacing="6" translatesAutoresizingMaskIntoConstraints="NO" id="tgj-yg-vqL">
<rect key="frame" x="81" y="46.5" width="233" height="73.5"/>
<subviews>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="classLocation" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="axi-2z-HNT">
<rect key="frame" x="0.0" y="0.0" width="233" height="20.5"/>
<color key="backgroundColor" red="0.55634254220000001" green="0.97934550050000002" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<nil key="textColor"/>
<nil key="highlightedColor"/>
</label>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="classDays" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="UK3-x3-XGT">
<rect key="frame" x="0.0" y="26.5" width="233" height="20.5"/>
<color key="backgroundColor" red="0.55634254220000001" green="0.97934550050000002" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<nil key="textColor"/>
<nil key="highlightedColor"/>
</label>
<label opaque="NO" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="classTime" textAlignment="natural" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" adjustsFontSizeToFit="NO" translatesAutoresizingMaskIntoConstraints="NO" id="tqR-li-CIc">
<rect key="frame" x="0.0" y="53" width="233" height="20.5"/>
<color key="backgroundColor" red="0.55634254220000001" green="0.97934550050000002" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<nil key="textColor"/>
<nil key="highlightedColor"/>
</label>
</subviews>
</stackView>
</subviews>
<constraints>
<constraint firstAttribute="bottomMargin" relation="greaterThanOrEqual" secondItem="tgj-yg-vqL" secondAttribute="bottom" constant="5" id="0xW-En-RuB"/>
<constraint firstItem="3yA-G1-vya" firstAttribute="top" secondItem="mVQ-Zs-26O" secondAttribute="bottom" constant="20" id="38Z-Q2-z0J"/>
<constraint firstItem="mVQ-Zs-26O" firstAttribute="leading" secondItem="G9i-wf-fpQ" secondAttribute="leadingMargin" constant="5" id="3J5-7X-0l3"/>
<constraint firstItem="cs2-jh-xoS" firstAttribute="leading" secondItem="tgj-yg-vqL" secondAttribute="trailing" constant="10" id="7TA-sg-Zod"/>
<constraint firstItem="mVQ-Zs-26O" firstAttribute="top" secondItem="G9i-wf-fpQ" secondAttribute="topMargin" constant="5" id="8Hh-cA-6Se"/>
<constraint firstItem="3yA-G1-vya" firstAttribute="leading" secondItem="G9i-wf-fpQ" secondAttribute="leadingMargin" constant="5" id="R99-Wc-e7A"/>
<constraint firstAttribute="trailingMargin" secondItem="cs2-jh-xoS" secondAttribute="trailing" constant="5" id="TZq-bM-V5Q"/>
<constraint firstAttribute="trailingMargin" secondItem="mVQ-Zs-26O" secondAttribute="trailing" constant="5" id="YaH-eA-zBR"/>
<constraint firstItem="cs2-jh-xoS" firstAttribute="top" secondItem="mVQ-Zs-26O" secondAttribute="bottom" constant="35" id="YcB-tX-G3j"/>
<constraint firstItem="tgj-yg-vqL" firstAttribute="top" secondItem="mVQ-Zs-26O" secondAttribute="bottom" constant="10" id="r6S-UG-b0o"/>
<constraint firstItem="tgj-yg-vqL" firstAttribute="leading" secondItem="3yA-G1-vya" secondAttribute="trailing" constant="10" id="xdc-Jv-acQ"/>
</constraints>
</tableViewCellContentView>
<connections>
<outlet property="classDays" destination="UK3-x3-XGT" id="3b4-tW-ATv"/>
<outlet property="classLocation" destination="axi-2z-HNT" id="59P-Dm-VIx"/>
<outlet property="className" destination="mVQ-Zs-26O" id="SaH-dm-Gxi"/>
<outlet property="classTime" destination="tqR-li-CIc" id="FNG-WI-vOC"/>
<outlet property="itemImageView" destination="cs2-jh-xoS" id="MKG-Dz-kFh"/>
<outlet property="starImageView" destination="3yA-G1-vya" id="dDq-hC-USx"/>
</connections>
</tableViewCell>
</prototypes>
<connections>
<outlet property="dataSource" destination="Rco-aF-G6p" id="Ear-AM-DTS"/>
<outlet property="delegate" destination="Rco-aF-G6p" id="DsX-fk-NBi"/>
</connections>
</tableView>
</tableViewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="ujd-EG-Zzl" userLabel="First Responder" customClass="UIResponder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="284" y="174.96251874062969"/>
</scene>
</scenes>
<resources>
<systemColor name="systemBackgroundColor">
<color white="1" alpha="1" colorSpace="custom" customColorSpace="genericGamma22GrayColorSpace"/>
</systemColor>
</resources>
</document>