This is minimal working example.
You have to use Tk()
only to create main window. For other windows you have to use Toplevel
. And when you have it in variable ie. top
then you have to use it as first argument in Label
(or other widget) to display widget in this window.
BTW: PEP 8 -- Style Guide for Python Code
#from tkinter import * # PEP8: `import *` is not preferred
import tkinter as tk
from PIL import ImageTk, Image
# --- functions ---
def click():
top = tk.Toplevel()
my_label = tk.Label(top, image=my_image)
my_label.pack()
# --- main ---
root = tk.Tk()
#root.configure(background="black") # PEP8: without spaces around `=`
my_image = ImageTk.PhotoImage(Image.open("lenna.png")) # PEP8: english names
my_button = tk.Button(root, text="Wyjście", command=click) # PEP8: lower_case_names for variables
my_button.pack()
root.mainloop()
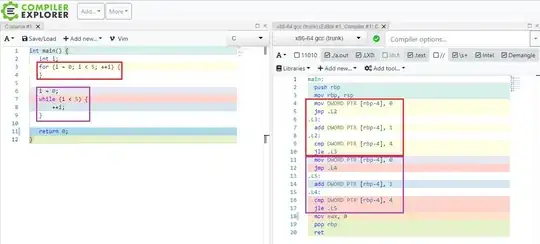
Image from Wikipedia: Lenna
EDIT:
I hope you didn't create PhotoImage
inside function because there is bug in PhotoImage
which removes image from memory when it is assigned to local variable in function. And then you can see empty image. It has to be assigned to global variable or to some object - ie. my_label.img = image