Here is a method of achieving the asked question. I'm using Angular (Typescript) but the principle remains the same.
Firstly you'll need these two methods / functions:
/**
* Returns the width of each item in the flexfox (they need to be equal width!)
*/
getFlexboxItemWidth(selector: string){
return document.querySelector(selector)?.getBoundingClientRect().width
}
/**
* Returns the amount of additional, invisible flexbox items to place
*/
calculateFlexboxFillerItemCount(totalItems: number, selector: string, flexboxSelector: string): number{
const flexboxWidth: number = document.querySelector(flexboxSelector)?.getBoundingClientRect().width as number
const itemsPerRow: number = Math.trunc(flexboxWidth / (this.getFlexboxItemWidth(selector) as number))
const itemsInLastIncompleteRow: number = totalItems % itemsPerRow
return isNaN(itemsPerRow)
|| isNaN(itemsInLastIncompleteRow)
|| itemsPerRow - itemsInLastIncompleteRow === Infinity
|| itemsInLastIncompleteRow === 0
? 0
: itemsPerRow - itemsInLastIncompleteRow + 1
}
Once you have created those two methods you'll need to implement them:
<!--The Flexbox-->
<ion-list id="home-tab--item-list-container" *ngIf="items.length > 0">
<!--Visible Flex Items-->
<ion-card class="home-tab--item-card" *ngFor="let item of items" (click)="goToItemDetails(item)">
<img [src]="item.images[0]">
<ion-card-header>
<ion-card-title>{{item.title}}</ion-card-title>
</ion-card-header>
<ion-card-content>
{{item.description}}
<div class="home-tab--item-slide-price">
${{item.cost.toFixed(2)}}
<div class="home-tab--item-rental-period" *ngIf="item.rentalPeriod !== null">
/{{item.rentalPeriod}}
</div>
</div>
</ion-card-content>
</ion-card>
<!--Invisible Flexbox Items responsible for filling remaining space of last row-->
<ion-card [ngStyle]="{width: getFlexboxItemWidth('.home-tab--item-card')+'px', visibility: 'hidden'}" *ngFor="let _ of [].constructor(calculateFlexboxFillerItemCount(items.length, '.home-tab--item-card', '#home-tab--item-list-container'))">
</ion-card>
</ion-list>
And finally, here is the styling for better context:
#home-tab--item-list-container {
display: flex;
justify-content: center;
flex-wrap: wrap;
width: 100%;
overflow: auto;
}
.home-tab--item-card {
min-width: 170px;
max-width: 300px;
cursor: pointer;
}
@media screen and ( max-width: 960px) {
.home-tab--item-card { flex: 0 1 30% }
}
End Result:
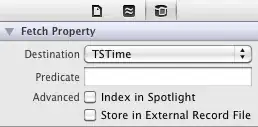