Your only option seems to be to draw the image to the canvas instead of creating a button (because widgets in Tkinter do not support transparency).
The drawback is, that you will have to check the mouse coordinates on the Canvas in order to see if your "Button" has been clicked.
The code would look like this:
from PIL import ImageTk, Image
root = Tk()
root.iconbitmap('unnamed.ico')
root.title('2048')
bg = ImageTk.PhotoImage(Image.open("welcome.png"))
new_game_btn = ImageTk.PhotoImage(Image.open("image_40.png"))
my_canvas = Canvas(root, width=780, height=550)
my_canvas.pack()
my_canvas.create_image(0, 0, image=bg, anchor=NW)
my_canvas.create_image(100, 100, image=new_game_btn, anchor=NW)
root.mainloop()
This results in that (don't mind the typo...):
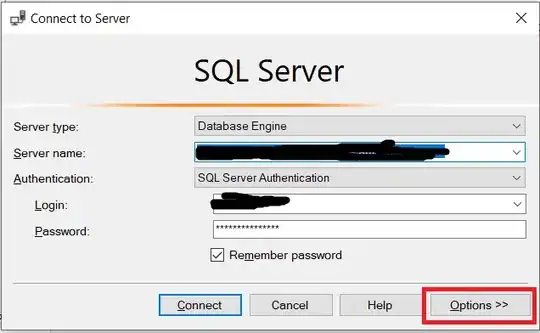
If you want to check if that part of the canvas was clicked, I would just check for the bounding box like this:
from tkinter import *
from PIL import ImageTk, Image
x_pos = 100
y_pos = 100
width = 100
height = 100
def callback(event):
print("Canvas clicked at", event.x, event.y)
if (event.x > x_pos and event.x < x_pos + width):
if(event.y > y_pos and event.y < y_pos + height):
print("The button was clicked (approximately).")
root = Tk()
root.iconbitmap('unnamed.ico')
root.title('2048')
bg = ImageTk.PhotoImage(Image.open("welcome.png"))
new_game_btn = ImageTk.PhotoImage(Image.open("image_40.png"))
my_canvas = Canvas(root, width=780, height=550)
my_canvas.pack()
my_canvas.create_image(0, 0, image=bg, anchor=NW)
my_canvas.create_image(100, 100, image=new_game_btn, anchor=NW)
my_canvas.bind("<Button-1>", callback)
root.mainloop()