Your problem is kinda similar to an application that I developed some time ago which also involved some LEDs and randomness.
I wrote the following code for running some tests before migrating the functionalities to the Arduino ecosystem.
Feel free to reuse and adapt my code to your needs. Keep in mind that I wrote it to be tested on C++17 using Codelite and not for the Arduino platform, therefore you can replace the random
function with the one from Arduino.
Hope it helps. If so, just show a bit of appreciation including the link to this answer in your code, for posterity ;)
#include <iostream>
#include <random>
#include <stdio.h>
#include <stdlib.h>
#include <string>
#include <time.h>
using namespace std;
#define MAX_COLORS 4
char textOut[100];
int cycles;
string colorNames[4] = { "RED", "BLUE", "GREEN", "PURPLE" };
typedef enum { RED, BLUE, GREEN, PURPLE } ColorList;
struct ColorsGroup {
ColorList colorCode;
string name;
};
ColorsGroup colorLED[4];
// Methods
int random(int, int);
ColorList retrieveColor(int);
void fillColors(void);
void printColors(int);
void setup()
{
fillColors();
cycles = 0;
}
int main()
{
cout << "********** Color picker *********" << endl;
setup();
while(cycles < 10) {
fillColors();
printColors(cycles);
cycles++;
}
return 0;
}
// From: https://stackoverflow.com/questions/7560114/random-number-c-in-some-range
int random(int min, int max)
{
static bool first = true;
if(first) {
srand(time(NULL));
first = false;
}
return min + rand() % ((max + 1) - min);
}
void fillColors(void)
{
for(int idx = 0; idx < MAX_COLORS; idx++) {
ColorList newColor = retrieveColor(random(0, MAX_COLORS - 1));
colorLED[idx].colorCode = newColor;
colorLED[idx].name = colorNames[newColor];
}
}
void printColors(int i)
{
sprintf(textOut, "%d. colorLED >> ", i);
cout << textOut;
for(int idx = 0; idx < MAX_COLORS; idx++) {
const char* nameStr = colorLED[idx].name.c_str(); // or &colorLED[idx].name[0];
sprintf(textOut, "%s[%d]", nameStr, colorLED[idx].colorCode);
cout << textOut;
if(idx <= MAX_COLORS - 2) {
sprintf(textOut, ", ");
cout << textOut;
}
else {
cout << ";" << endl;
}
}
}
ColorList retrieveColor(int col)
{
switch(col) {
case 0:
return RED;
break;
case 1:
return BLUE;
break;
case 2:
return GREEN;
break;
case 3:
return PURPLE;
break;
default:
return RED; // for the sake of completeness
break;
}
}
And this code spits out the following:
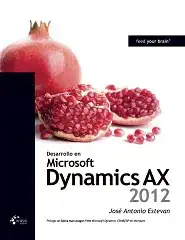