So, to wrap-up the requirements:
- Removing the three dots icon from the top. Instead, having a bottom button that shows the overflow options menu.
- Anchor the options overflow menu to the bottom (instead of the top) when the bottom button is hit.
The system overflow options menu should be anchored to the actionBar/toolBar that hosts it; and therefore you can't show this menu at bottom unless you move this bar to the bottom too. Otherwise you need to create a customized popup menu rather than the system one.
So, the solution here is to create a customized Toolbar
at the bottom of the activity:
Layout:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.google.android.material.appbar.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"
app:layout_constraintBottom_toBottomOf="parent">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:popupTheme="@style/ThemeOverlay.AppCompat.Light" />
</com.google.android.material.appbar.AppBarLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
And this requires to use a NoActionBar
theme at themes.xml
or styles.xml
such as:
Theme.MaterialComponents.DayNight.NoActionBar
or Theme.AppCompat.DayNight.NoActionBar
.
And set the new supportActionBar
in behavior:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val toolbar = findViewById<Toolbar>(R.id.toolbar)
setSupportActionBar(toolbar)
title = ""
}
override fun onCreateOptionsMenu(menu: Menu): Boolean {
menuInflater.inflate(R.menu.menu_main, menu)
return true
}
}
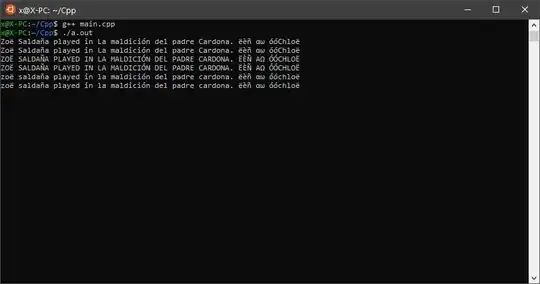
This fulfills the top mentioned requirements apart from that the new supportActionBar
is now at the bottom, so you probably need to add another toolbar at the top to be customized as you want.
In order to replace the three-dots icon at the bottom with a gauge icon, there are multiple answers here, and here is a one:
Add the below style:
<style name="customoverflow">
<item name="android:src">@drawable/ic_baseline_settings_24</item>
</style>
Apply it to the main theme:
<item name="android:actionOverflowButtonStyle">@style/customoverflow</item>
You can also add a paddingEnd
to the toolBar
to have some space between the icon and the far end of the screen:
<androidx.appcompat.widget.Toolbar
...
android:paddingEnd="16dp"
android:paddingRight="16dp"/>
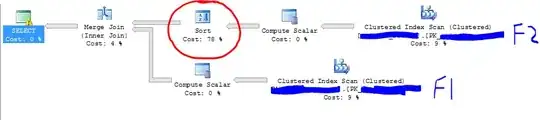