So I found this article https://medium.com/@pinkesh.earth/flutter-quick-tip-how-to-set-text-background-color-with-curve-d40a2f96a415
It describes how to use textstyle to look like what you want, but it doesn't exactly work like that, I don't know why. It draws the next line's background above the previous.
I managed to work around it (bug?) by stacking two textfields(one has transparent text, the other transparent background).
The result:
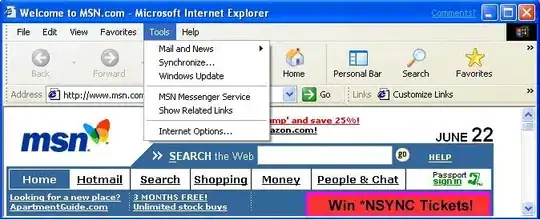
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
final myControllerName = TextEditingController();
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
inputDecorationTheme: const InputDecorationTheme(
fillColor: Colors.transparent,
filled: true,
focusedBorder: UnderlineInputBorder(
borderSide: BorderSide(color: Colors.transparent)),
enabledBorder: UnderlineInputBorder(
borderSide: BorderSide(color: Colors.transparent),
),
border: UnderlineInputBorder(
borderSide: BorderSide(color: Colors.transparent),
),
)),
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: Stack(
children: [
IntrinsicWidth(
child: TextField(
controller: myControllerName,
style: TextStyle(
color: Colors.transparent,
fontWeight: FontWeight.w600,
fontSize: 20,
background: Paint()
..strokeWidth = 25
..color = Colors.blue
..style = PaintingStyle.stroke
..strokeJoin = StrokeJoin.round),
keyboardType: TextInputType.multiline,
maxLines: null,
textAlign: TextAlign.center,
),
),
IntrinsicWidth(
child: TextField(
controller: myControllerName,
style: const TextStyle(
color: Colors.white,
fontWeight: FontWeight.w600,
fontSize: 20,
backgroundColor: Colors.transparent),
keyboardType: TextInputType.multiline,
maxLines: null,
textAlign: TextAlign.center,
),
)
],
))));
}
}