As you wish to be able to read/write to this file, you should keep it external from your code. This means that your BufferedReader
will have to be created from a filesystem path. Now there are several ways you could that, but you should preferably use java.nio
API rather than the older java.io.File
.
I can see mainly 3 scenarios here:
- Use a relative path from working directory
.
, that is your project's location (NOT src
) when debuging from IDE, or right next to your jar once it has been made:
try (BufferedReader br = Files.newBufferedReader(Paths.get("./test.txt"), StandardCharsets.UTF_8)) {
//Do your stuff
}
Or if your file's in [projectDir]/pingus
(still NOT src
)
try (BufferedReader br = Files.newBufferedReader(Paths.get("./pingus/test.txt"), StandardCharsets.UTF_8)) {
//Do your stuff
}
Note it will depend on where your application was launched from, but it's generally fine.
- Use an absolute path generated from your code's storage location:
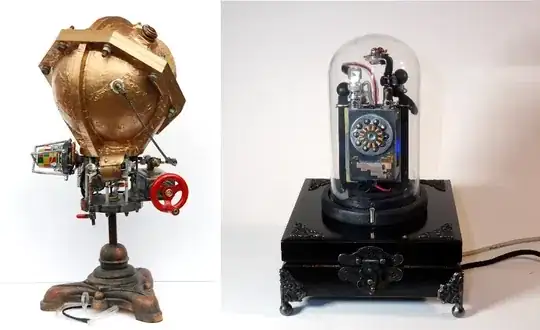
package pingus;
import java.io.BufferedReader;
import java.io.IOException;
import java.net.URISyntaxException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Pongus {
public static void main(String[] args) throws URISyntaxException, IOException {
//Find codebase directory
Path codebase = Paths.get(Pongus.class.getProtectionDomain().getCodeSource().getLocation().toURI());
Path absdir = codebase.getParent().normalize().toAbsolutePath();
System.out.println("Working from " + absdir);
//Path to your file
Path txtFilePath = absdir.resolve("pingus/test.txt");
System.out.println("File path " + txtFilePath);
//Read
try (BufferedReader rdr = Files.newBufferedReader(txtFilePath, StandardCharsets.UTF_8)) {
System.out.println("Printing first line:");
System.out.println("\t" + rdr.readLine());
}
}
}
Note this will definitely work when running from a jar. It will work during debugging using Eclipse in its default configuration, as codebase
will initially point to the bin
sub-folder in your project's path. It might not work with other IDE configurations where your compiled class can be stored elsewhere
- Use an absolute path on your filesystem, either hard-coded or from some configuration.
try (BufferedReader rdr = Files.newBufferedReader(Paths.get("C:\\mydirs\\pingus\\test.txt"), StandardCharsets.UTF_8)) {
//Do your stuff
}