You could add wkhtmltopdf
to your exe
using PyInstaller
:
import subprocess
import sys
htmlPath = 'C:/temp/test.html'
pdfPath = 'C:/temp/test_through_exe.pdf'
if getattr(sys, 'frozen', False):
# Change wkhtmltopdf path for exe!
wkPath = os.path.join(sys._MEIPASS, "wkhtmltopdf.exe")
else:
wkPath = 'C:/.../Downloads/wkhtmltopdf.exe'
with open(htmlPath, 'w') as f:
f.write('<html><body><h1>%s</h1></body></html>' % sys.version)
cmd = '%s %s %s' % (wkPath, htmlPath, pdfPath)
print(cmd)
proc = subprocess.Popen(cmd, stderr=subprocess.PIPE, stdout=subprocess.PIPE, shell=True)
stdout, stderr = proc.communicate()
print(proc.returncode, stdout)
print(proc.returncode, stderr)
Building the exe
(wkhtmltopdf and your script in the same directory):
PyInstaller --onefile --add-data ./wkhtmltopdf.exe;. test.py
Out:
C:\Users\xxx\AppData\Local\Temp\_MEI33842\wkhtmltopdf.exe C:/temp/test.html C:/temp/test_through_exe.pdf
0 b''
0 b'Loading pages (1/6)\r\n[> ] 0%\r[======>
] 10%\r[==============================> ] 50%\r[============================================================] 100%\rCounting pages (2/6) \r\n[============================================================] Object 1 of 1\rResolving links (4/6) \r\n[============================================================] Object 1 of 1\rLoading headers and footers (5/6) \r\nPrinting pages (6/6)\r\n[>
] Preparing\r[============================================================] Page 1 of 1\rDone
\r\n'
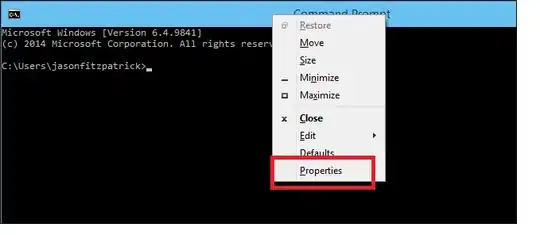