Runnable example based on Leon Shpaner's answer:
import scipy as sp
import seaborn as sns
import matplotlib
import matplotlib.pyplot as plt
matplotlib.use('Agg')
sns.set_theme(style="ticks")
x_data = [1,2,3,4,5,6,7,8,9]
y_data = [1,3,2,4,5,6,7,9,8]
sns.scatterplot(x=x_data, y=y_data)
plt.title('Title')
plt.xlabel('x-axis label')
plt.ylabel('y-axis label')
r, p = sp.stats.pearsonr(x=x_data, y=y_data)
ax = plt.gca() # Get a matplotlib's axes instance
plt.text(.05, .8, "Pearson's r ={:.2f}".format(r), transform=ax.transAxes)
plt.savefig('Scatterplot with Pearson r.png', bbox_inches='tight', dpi=300)
plt.close()
outputs:

If one wants to also add the correlation line:
import scipy as sp
import seaborn as sns
import matplotlib
import matplotlib.pyplot as plt
matplotlib.use('Agg')
sns.set_theme(style="ticks")
x_data = [1,2,3,4,5,6,7,8,9]
y_data = [1,3,2,4,5,6,7,9,8]
sns.scatterplot(x=x_data, y=y_data)
plt.title('Title')
plt.xlabel('x-axis label')
plt.ylabel('y-axis label')
r, p = sp.stats.pearsonr(x=x_data, y=y_data)
ax = plt.gca() # Get a matplotlib's axes instance
plt.text(.05, .8, "Pearson's r ={:.2f}".format(r), transform=ax.transAxes)
# The following code block adds the correlation line:
import numpy as np
m, b = np.polyfit(x_data, y_data, 1)
X_plot = np.linspace(ax.get_xlim()[0],ax.get_xlim()[1],100)
plt.plot(X_plot, m*X_plot + b, '-')
plt.savefig('Scatterplot with Pearson r.png', bbox_inches='tight', dpi=300)
plt.close()
outputs:
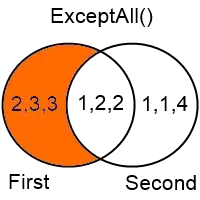
Related, still in Python but without seaborn
: How to overplot a line on a scatter plot in python?