I know this is an old post but might help someone in the future.
Disclaimer: My solution is a workaround that's a little clunky and not sexy, but it works. I tried to find a proper solution by even going into the Vista themes' .tcl file but apparently, the style of the widgets themselves is given through the Microsoft Visual Styles API. I tried playing around with it but it seems that fixing the issue in the themes' code could be deeper than my current understanding of the tcl language.
Workaround I found:
Instead of setting the master of the Scale to be optionsframe
, create another frame to contain the Scale and a variable to store the height of the frame (this will come in handy later):
scale_frame_height = 30
scale_frame = ttk.Frame(master=optionsframe, height=scale_frame_height)
scale_frame.grid(row=0, column=7)
Pack the scale into the container frame:
width = Scale(scale_frame, from_ = 1, to = 20, style = 'SCALEBG.Horizontal.TScale')
width.pack()
Now the "cheat" is to create another frame with the same height as the scale_frame
and a width of 2 pixels and place it into the scale_frame
container:
hide_pixel_frame = ttk.Frame(master=scale_frame, height=scale_frame_height, width=2)
hide_pixel_frame.place(relx=1, rely=0, anchor="ne")
The hide_pixel_frame
will be laid over the excess pixels and always stick to the right:
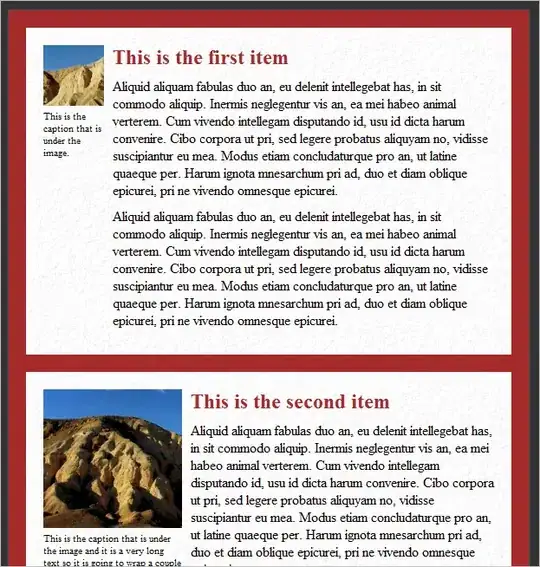
Now create a style for the hide_pixel_frame to give it the same background color as the window and add the style to the frame:
hide_pixel_bg = ttk.Style()
hide_pixel_bg.configure('HIDE.TFrame', foreground = '#2a2a2a', background = '#2a2a2a')
hide_pixel_frame = ttk.Frame(master=scale_frame, height=scale_frame_height, width=2, style='HIDE.TFrame')
hide_pixel_frame.place(relx=1, rely=0, anchor="ne")
The final example code would look like this:
import tkinter as tk
from tkinter import ttk
from tkinter.ttk import Scale
root = tk.Tk()
style = ttk.Style(root)
style.theme_use("vista")
root.configure(bg='#2a2a2a')
scalebg = ttk.Style()
scalebg.configure('SCALEBG.Horizontal.TScale', foreground = '#2a2a2a', background = '#2a2a2a')
optionsframe = ttk.Frame(master=root, width=300, height=50)
optionsframe.pack_propagate()
optionsframe.pack(pady=30)
scale_frame_height = 30
scale_frame = ttk.Frame(master=optionsframe, height=scale_frame_height)
scale_frame.grid(row=0, column=7)
width = Scale(scale_frame, from_ = 1, to = 20, style = 'SCALEBG.Horizontal.TScale')
width.pack()
hide_pixel_bg = ttk.Style()
hide_pixel_bg.configure('HIDE.TFrame', foreground = '#2a2a2a', background = '#2a2a2a')
hide_pixel_frame = ttk.Frame(master=scale_frame, height=scale_frame_height, width=2, style='HIDE.TFrame')
hide_pixel_frame.place(relx=1, rely=0, anchor="ne")
root.mainloop()
The end result: 
Again, this is quite a crude workaround but it's better than nothing and it gets rid of the pesky pixels.