When the user starts typing into the entry field, I want the
possibilities shown underneath the tkinter entry field just as if you
would type into the Google search bar.
How can I make this entry field act the same as the Google search bar.
The solution can be fixed.
- Add
Entry
widget.
- Add
Listbox
widget.
- Create
check_key()
function. This will search in Listbox
for what you type.
- Add
e.bind
for check_key()
function. We'll be using Walrus.
- Create
update()
function. It will remove item when pressing the
spacebar to update the Listbox.
- Create
items_selected()
function. If you desire, then click the
item on Listbox to update the Entry widget.
- Add
lb.bind
for items_selected()
function.
Snippet:
import tkinter as tk
root = tk.Tk()
root.title('Search Engines')
#fruits = ["Apple", "Banana", "Peach", "Pear"]
lst = ['san francisco weather', 'san francisco', 'san francisco giants',
'san francisco valleyr', 'san francisco state university',
'san francisco hotels', 'san francisco 49ers', 'san fernando',
'san francisco mission', 'san francisco zip code']
def check_key(event: bool) -> bool:
if (value := event.widget.get()) =='':
data = lst
else:
data = [item for item in lst if value.lower() in item.lower()]
update(data)
def update(data: str) -> None:
lb.delete(0, 'end')
for item in data:
lb.insert('end', item)
def items_selected(event: None) -> None:
selected_langs = ",".join([lb.get(i) for i in lb.curselection()])
e.delete(0, tk.END)
e.insert(0, selected_langs)
e = tk.Entry(root)
lb = tk.Listbox(root)
e.pack()
lb.pack()
e.bind('<KeyRelease>', check_key)
lb.bind('<<ListboxSelect>>', items_selected)
update(lst)
root.mainloop()
Screenshot:
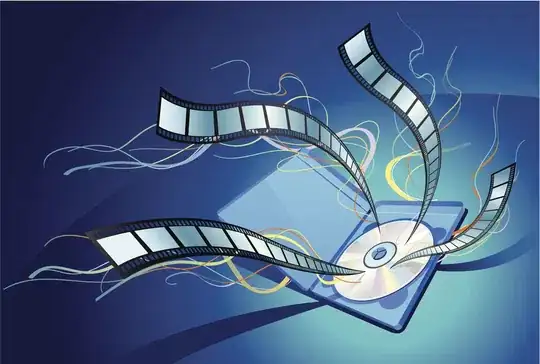