Unfortunately, you can't make client requests to https://www.youtube.com and avoid CORS policy, because it is a built-in system on browser for request rejecting someones like you =)
But.
We can avoid CORS policy by making proxy server side request and pretending as client. After that, you can move all logic to your server.
- Create
index.js
file on your localhost with next content
// Import the required http module
const http = require('http');
const https = require('https');
const url = require('url');
function youtubeRequest(channelId, headers) {
return new Promise((resolve, reject) => {
const options = {
hostname: 'www.youtube.com',
path: `/channel/${channelId}`,
method: 'GET',
headers: headers
};
let raw = ''
const req = https.request(options, (res) => {
res.on('data', (d) => {
raw += d;
});
res.on('end', () => {
resolve(raw);
});
});
req.on('error', (error) => {
console.log(error);
reject(error);
});
req.end();
});
}
// Define the request handler function
const requestHandler = async (req, res) => {
const parsedUrl = url.parse(req.url, true);
// Check if the request method is GET and the path is '/call'
if (req.method === 'GET' && parsedUrl.pathname === '/isLive') {
const channelId = parsedUrl.query.channelId;
if (!channelId) {
res.writeHead(404, { 'Content-Type': 'application/json' });
const jsonResponse = { message: 'Channel id is invalid' };
res.end(JSON.stringify(jsonResponse));
return;
}
try {
const HTML = await youtubeRequest(channelId, {
"accept": "text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7",
"accept-language": "en,ru-RU;q=0.9,ru;q=0.8,en-US;q=0.7",
"cache-control": "max-age=0",
});
let isLive = false;
if (HTML.includes("hqdefault_live.jpg")) {
isLive = true;
}
res.writeHead(200, {
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Headers": "Origin, X-Requested-With, Content-Type, Accept",
'Content-Type': 'application/json'
});
// Send a JSON response
const jsonResponse = {
isLive,
message: isLive ? 'Your channel is live' : 'Your channel disabled'
};
res.end(JSON.stringify(jsonResponse));
} catch (e) {
console.log(e);
res.writeHead(500, { 'Content-Type': 'application/json' });
// Send a JSON response
const jsonResponse = { message: 'Something went wrong, check your server logs' };
res.end(JSON.stringify(jsonResponse));
}
} else {
// Send a 404 response for other routes
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('Not found');
}
};
// Create the server with the request handler
const server = http.createServer(requestHandler);
// Start the server listening on a specified port
const port = 3000;
server.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
- Start it with bash command
node index.js
. If you havent install NodeJs you can do with official webpage.
After that, your server should be running at http://localhost:3000
- Now, you can make client request for
http://localhost:3000/isLive?channelId=UCnB-Fhp5FQfCZNfdAvm27Qw
So, use below client javascript for calling your web server
<script>
var channelid = "UCnB-Fhp5FQfCZNfdAvm27Qw"; // REPLACE WITH YOUR CHANNEL ID
fetch('http://localhost:3000/isLive?channelId=' + channelid).then(function (response) {
return response.text();
}).then(function (response) {
console.log(response);
}).catch(function (err) {
console.warn('Something went wrong', err);
});
</script>
Now, in browser console, you can see result
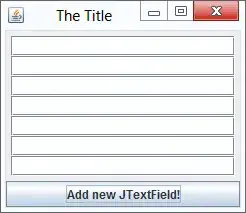