I want to return value without "bag", "shirts","shoes". Look like
above:
Well, based on your question, you could achieve by introduing new model which would be containing all property that your "bag", "shirts","shoes" containing. Finally, you need to loop through the existing list item and bind those into new class/model. You can have a try in following way:
Create A new Model for generic List:
public class GenericClass
{
public int Id { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
public string Category { get; set; }
}
Note: We will use this model to loop over our existing list thus we would bind them into this.
Base Model:
public class GenericClass
{
public int Id { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
public string Category { get; set; }
}
public class Bags
{
public int Id { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
public string Category { get; set; }
}
public class Shirts
{
public int Id { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
public string Category { get; set; }
}
public class Shoes
{
public int Id { get; set; }
public string Name { get; set; }
public int Quantity { get; set; }
public string Category { get; set; }
}
Seed Data Into Model:
List<Bags> listBags = new List<Bags>();
listBags.Add(new Bags() { Id = 101, Name = "Bag A", Quantity = 10, Category = "Cat-A" });
listBags.Add(new Bags() { Id = 102, Name = "Bag B", Quantity = 15, Category = "Cat-A" });
listBags.Add(new Bags() { Id = 103, Name = "Bag C", Quantity = 20, Category = "Cat-A" });
List<Shirts> listShirts = new List<Shirts>();
listShirts.Add(new Shirts() { Id = 101, Name = "Shirt A", Quantity = 10, Category = "Cat-B" });
listShirts.Add(new Shirts() { Id = 102, Name = "Shirt B", Quantity = 15, Category = "Cat-B" });
listShirts.Add(new Shirts() { Id = 103, Name = "Shirt C", Quantity = 20, Category = "Cat-B" });
List<Shoes> listShoes = new List<Shoes>();
listShoes.Add(new Shoes() { Id = 101, Name = "Shirt A", Quantity = 10, Category = "Cat-S" });
listShoes.Add(new Shoes() { Id = 102, Name = "Shirt B", Quantity = 15, Category = "Cat-S" });
listShoes.Add(new Shoes() { Id = 103, Name = "Shirt C", Quantity = 20, Category = "Cat-S" });
Build Custom List:
var genericClass = new List<GenericClass>();
foreach (var item in listBags)
{
var bag = new GenericClass();
bag.Id = item.Id;
bag.Name = item.Name;
bag.Quantity = item.Quantity;
bag.Category = item.Category;
genericClass.Add(bag);
}
foreach (var item in listShirts)
{
var shirt = new GenericClass();
shirt.Id = item.Id;
shirt.Name = item.Name;
shirt.Quantity = item.Quantity;
shirt.Category = item.Category;
genericClass.Add(shirt);
}
foreach (var item in listShoes)
{
var shoes = new GenericClass();
shoes.Id = item.Id;
shoes.Name = item.Name;
shoes.Quantity = item.Quantity;
shoes.Category = item.Category;
genericClass.Add(shoes);
}
Complete Demo:
[HttpGet("GetFrom3TablesWithSameKey")]
public IActionResult GetFrom3TablesWithSameKey()
{
List<Bags> listBags = new List<Bags>();
listBags.Add(new Bags() { Id = 101, Name = "Bag A", Quantity = 10, Category = "Cat-A" });
listBags.Add(new Bags() { Id = 102, Name = "Bag B", Quantity = 15, Category = "Cat-A" });
listBags.Add(new Bags() { Id = 103, Name = "Bag C", Quantity = 20, Category = "Cat-A" });
List<Shirts> listShirts = new List<Shirts>();
listShirts.Add(new Shirts() { Id = 101, Name = "Shirt A", Quantity = 10, Category = "Cat-B" });
listShirts.Add(new Shirts() { Id = 102, Name = "Shirt B", Quantity = 15, Category = "Cat-B" });
listShirts.Add(new Shirts() { Id = 103, Name = "Shirt C", Quantity = 20, Category = "Cat-B" });
List<Shoes> listShoes = new List<Shoes>();
listShoes.Add(new Shoes() { Id = 101, Name = "Shirt A", Quantity = 10, Category = "Cat-S" });
listShoes.Add(new Shoes() { Id = 102, Name = "Shirt B", Quantity = 15, Category = "Cat-S" });
listShoes.Add(new Shoes() { Id = 103, Name = "Shirt C", Quantity = 20, Category = "Cat-S" });
var genericClass = new List<GenericClass>();
foreach (var item in listBags)
{
var bag = new GenericClass();
bag.Id = item.Id;
bag.Name = item.Name;
bag.Quantity = item.Quantity;
bag.Category = item.Category;
genericClass.Add(bag);
}
foreach (var item in listShirts)
{
var shirt = new GenericClass();
shirt.Id = item.Id;
shirt.Name = item.Name;
shirt.Quantity = item.Quantity;
shirt.Category = item.Category;
genericClass.Add(shirt);
}
foreach (var item in listShoes)
{
var shoes = new GenericClass();
shoes.Id = item.Id;
shoes.Name = item.Name;
shoes.Quantity = item.Quantity;
shoes.Category = item.Category;
genericClass.Add(shoes);
}
return Ok(genericClass);
}
Output:
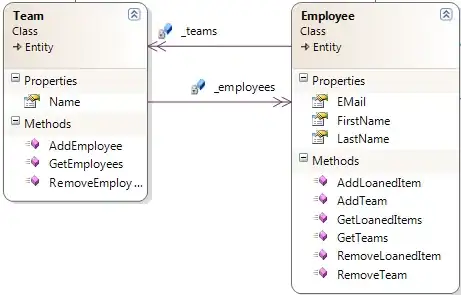
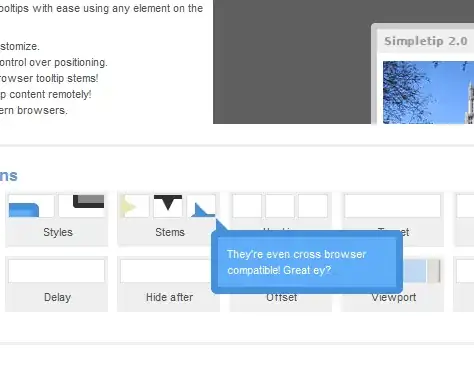
Note: If you still have any concern please have a look on our official documnet here.