There are multiple ways of achieving that, it is described in the "Arranging multiple Axes in a Figure" part of the matplotlib documentation.
Below is a basic recopy for your case:
import matplotlib.pyplot as plt
figsize = (7, 4)
# Method 1
fig, axd = plt.subplot_mosaic(
[
["1", "2", "3"],
["1", "2", "4"],
],
figsize=figsize,
layout="constrained",
)
# Method 2
fig = plt.figure(figsize=figsize, layout="constrained")
spec = fig.add_gridspec(ncols=3, nrows=2)
ax1 = fig.add_subplot(spec[:, 0])
ax2 = fig.add_subplot(spec[:, 1])
ax3 = fig.add_subplot(spec[0, 2])
ax4 = fig.add_subplot(spec[1, 2])
# Method 3
fig = plt.figure(figsize=figsize, layout="constrained")
spec0 = fig.add_gridspec(ncols=2, nrows=1, width_ratios=[2, 1])
spec01 = spec0[0].subgridspec(ncols=2, nrows=1)
spec02 = spec0[1].subgridspec(ncols=1, nrows=2)
ax1 = fig.add_subplot(spec01[0])
ax2 = fig.add_subplot(spec01[1])
ax3 = fig.add_subplot(spec02[0])
ax4 = fig.add_subplot(spec02[1])
plt.show()
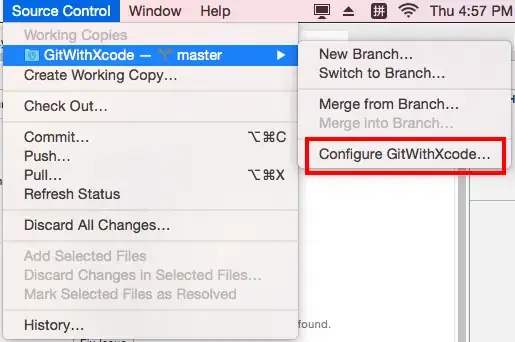