In case you're making custom back button basing on UIButton with image of arrow, here is the subclass snippet.
Using it you can either create button in code or just assign class in Interface Builder to any UIButton.
Back Arrow Image will be added automatically and colored with text color.
@interface UIImage (TintColor)
- (UIImage *)imageWithOverlayColor:(UIColor *)color;
@end
@implementation UIImage (TintColor)
- (UIImage *)imageWithOverlayColor:(UIColor *)color
{
CGRect rect = CGRectMake(0.0f, 0.0f, self.size.width, self.size.height);
if (UIGraphicsBeginImageContextWithOptions) {
CGFloat imageScale = 1.0f;
if ([self respondsToSelector:@selector(scale)])
imageScale = self.scale;
UIGraphicsBeginImageContextWithOptions(self.size, NO, imageScale);
}
else {
UIGraphicsBeginImageContext(self.size);
}
[self drawInRect:rect];
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextSetBlendMode(context, kCGBlendModeSourceIn);
CGContextSetFillColorWithColor(context, color.CGColor);
CGContextFillRect(context, rect);
UIImage *image = UIGraphicsGetImageFromCurrentImageContext();
UIGraphicsEndImageContext();
return image;
}
@end
#import "iOS7backButton.h"
@implementation iOS7BackButton
-(void)awakeFromNib
{
[super awakeFromNib];
BOOL is6=([[[UIDevice currentDevice] systemVersion] floatValue] <7);
UIImage *backBtnImage = [[UIImage imageNamed:@"backArrow"] imageWithOverlayColor:self.titleLabel.textColor];
[self setImage:backBtnImage forState:UIControlStateNormal];
[self setTitleEdgeInsets:UIEdgeInsetsMake(0, 5, 0, 0)];
[self setImageEdgeInsets:UIEdgeInsetsMake(0, is6?0:-10, 0, 0)];
}
+ (UIButton*) buttonWithTitle:(NSString*)btnTitle andTintColor:(UIColor*)color {
BOOL is6=([[[UIDevice currentDevice] systemVersion] floatValue] <7);
UIButton *backBtn=[[UIButton alloc] initWithFrame:CGRectMake(0, 0, 60, 30)];
UIImage *backBtnImage = [[UIImage imageNamed:@"backArrow"] imageWithOverlayColor:color];
[backBtn setImage:backBtnImage forState:UIControlStateNormal];
[backBtn setTitleEdgeInsets:UIEdgeInsetsMake(0, is6?5:-5, 0, 0)];
[backBtn setImageEdgeInsets:UIEdgeInsetsMake(0, is6?0:-10, 0, 0)];
[backBtn setTitle:btnTitle forState:UIControlStateNormal];
[backBtn setTitleColor:color /*#007aff*/ forState:UIControlStateNormal];
return backBtn;
}
@end
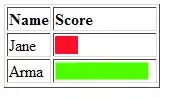