Look dude, I made a simple "File Explorer" example in a couple of lines of XAML in 5 minutes.
There is NO need to create your own ListViewItem
or anything like that. WPF is NOT winforms. You need to understand this.
I already explained this to you. UI is Not Data.
Look:
<Window x:Class="WpfApplication14.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication14"
Title="MainWindow" Height="350" Width="525">
<ListView ItemsSource="{Binding}">
<ListView.ItemContainerStyle>
<Style TargetType="ListViewItem">
<Setter Property="IsSelected" Value="{Binding IsSelected}"/>
</Style>
</ListView.ItemContainerStyle>
<ListView.View>
<GridView>
<GridViewColumn>
<GridViewColumn.CellTemplate>
<DataTemplate>
<CheckBox IsChecked="{Binding IsSelected}"/>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
<GridViewColumn Header="Name" DisplayMemberBinding="{Binding Name}"/>
<GridViewColumn Header="Size">
<GridViewColumn.CellTemplate>
<DataTemplate>
<TextBlock x:Name="Size" Text="{Binding Size}"/>
<DataTemplate.Triggers>
<DataTrigger Binding="{Binding IsFile}" Value="False">
<Setter TargetName="Size" Property="Text" Value="[Directory]"/>
</DataTrigger>
</DataTemplate.Triggers>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
<GridViewColumn Header="Path" DisplayMemberBinding="{Binding Path}"/>
</GridView>
</ListView.View>
</ListView>
</Window>
Code Behind:
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
var path = @"C:\";
var dirs = Directory.GetDirectories(path)
.Select(x => new DirectoryInfo(x))
.Select(x => new FileViewModel()
{
Name = x.Name,
Path = x.FullName,
IsFile = false,
});
var files = Directory.GetFiles(path)
.Select(x => new FileInfo(x))
.Select(x => new FileViewModel()
{
Name = x.Name,
Path = x.FullName,
Size = x.Length,
IsFile = true,
});
DataContext = dirs.Concat(files).ToList();
}
}
Data Item:
public class FileViewModel: INotifyPropertyChanged
{
private bool _isSelected;
public bool IsSelected
{
get { return _isSelected; }
set
{
_isSelected = value;
OnPropertyChanged("IsSelected");
}
}
public string Name { get; set; }
public long Size { get; set; }
public string Path { get; set; }
public bool IsFile { get; set; }
public ImageSource Image { get; set; }
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null) handler(this, new PropertyChangedEventArgs(propertyName));
}
}
Result:
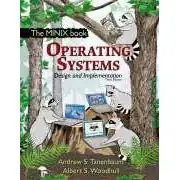
Do you see? There is no need to overcomplicate anything. WPF has everything you need to do anything.