MainViewModel Code:
namespace Question48240657
{
public class MainViewModel
{
public List<object> Groups { get; set; }
public List<Data> MainData { get; set; }
public MainViewModel()
{
MainData = GetData();
Groups = GetGroups(MainData);
}
private List<object> GetGroups(List<Data> mainData)
{
var groups = new List<object>()
{
new Group1() { DataList = mainData.Where(x => x.GropuName == "Group1").ToList() },
new Group2() { DataList = mainData.Where(x => x.GropuName == "Group1").ToList() },
new Group3() { DataList = mainData.Where(x => x.GropuName == "Group1").ToList() },
};
return groups;
}
public List<Data> GetData()
{
var dataList = new List<Data>()
{
new Data() { Name = "Data1", GropuName = "Group1" },
new Data() { Name = "Data2", GropuName = "Group1" },
new Data() { Name = "Data3", GropuName = "Group1" },
new Data() { Name = "Data4", GropuName = "Group2" },
new Data() { Name = "Data5", GropuName = "Group2" },
new Data() { Name = "Data6", GropuName = "Group2" },
new Data() { Name = "Data7", GropuName = "Group3" },
new Data() { Name = "Data8", GropuName = "Group3" },
new Data() { Name = "Data9", GropuName = "Group3" },
};
return dataList;
}
}
#region Classes from directory "Model"
public class Data
{
public string Name { get; set; }
public string GropuName { get; set; }
}
public class Group1
{
public List<Data> DataList { get; set; }
}
public class Group2
{
public List<Data> DataList { get; set; }
}
public class Group3
{
public List<Data> DataList { get; set; }
}
#endregion
}
Xaml Code:
<Window x:Class="Question48240657.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:Question48240657"
mc:Ignorable="d"
Title="MainWindow" Height="350" Width="525">
<Window.DataContext>
<local:MainViewModel/>
</Window.DataContext>
<Window.Resources>
<DataTemplate DataType="{x:Type local:Group1}">
<TabControl ItemsSource="{Binding DataList}" DisplayMemberPath="Name"/>
</DataTemplate>
<DataTemplate DataType="{x:Type local:Group2}">
<ListBox ItemsSource="{Binding DataList}" DisplayMemberPath="Name"/>
</DataTemplate>
<DataTemplate DataType="{x:Type local:Group3}">
<ItemsControl ItemsSource="{Binding DataList}" DisplayMemberPath="Name">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<WrapPanel/>
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
</ItemsControl>
</DataTemplate>
</Window.Resources>
<Grid>
<ItemsControl ItemsSource="{Binding Groups}"/>
</Grid>
</Window>
Example Image
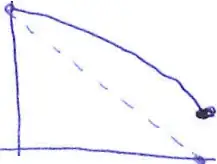