This is probably what you're looking for: changing hue
But if you for some reason don't want to use numpy, you could play around with composite and the alpha channels (the below puts an alpha value across the entire image, you could calculate via the green/red color of img1 where to change the alpha of img2):
from PIL import Image, ImageEnhance
img1 = Image.open('apple.png')
img2 = Image.open('green.png')
img2.putalpha(ImageEnhance.Brightness(img2.split()[3]).enhance(0.75))
img1 = Image.composite(img2, img1, img2)
img1.save('out.png')
Here are the two images (al tho a bit large perhaps) I used for the above test, and the third image is the result of the above code:
apple.png
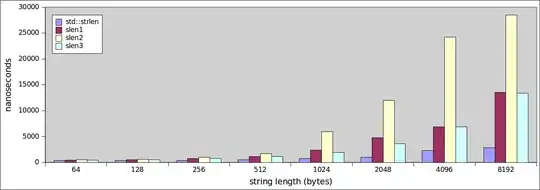
green.png
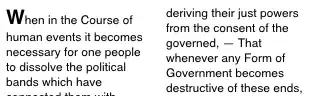
out.png
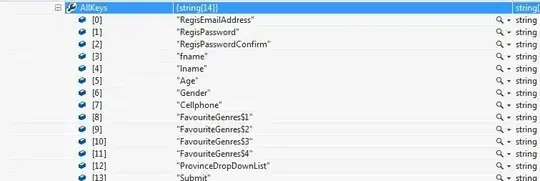
There's also PIL's paste()
function:
from PIL import Image, ImageEnhance
img = Image.open('greenapple.png', 'r')
img_w, img_h = img.size
red = Image.open('redcolor.png', 'r')
# red_w, red_h = red.size
new = Image.new('RGBA', (1024,769), (255, 255, 255, 255))
new_w, new_h = new.size
offset=((new_w-img_w)/2,(new_h-img_h)/2)
red.putalpha(ImageEnhance.Brightness(red.split()[3]).enhance(0.75))
new.paste(img, offset)
new.paste(red, offset)
new.save('out.png')
Play around with img.split()
which gives you red, green, blue, alpha
and use the green/red color patches when determining where to put the overlay.
Here are some more calculated alternatives where you for instance can use black as the exclusion color: