You con use this Answer to draw arc.
To find center for arc.
You can use Google Geometry library to find length of chord between 2 markers,its bearing and position of the cente of arc.
From documentation
computeDistanceBetween(from:LatLng, to:LatLng, radius?:number) Returns
the distance between two LatLngs as a number in meters. The radius
defaults to the Earth's radius in meters(6378137).
computeHeading(from:LatLng, to:LatLng) Returns the heading from one
LatLng to another LatLng. Headings are expressed in degrees clockwise
from North within the range [-180,180) as a number.
computeOffset(from:LatLng, distance:number, heading:number,
radius?:number) Returns the LatLng resulting from moving a distance
from an origin in the specified heading (expressed in degrees
clockwise from north) as LatLng.
First find distance between 2 markers
var spherical = google.maps.geometry.spherical;
var point1 = markers[0].getPosition();
var point2 = markers[1].getPosition();
var length = google.maps.geometry.spherical.computeDistanceBetween(point1,point2);
Then find bearing
var heading = google.maps.geometry.spherical.computeHeading(point1,point2);
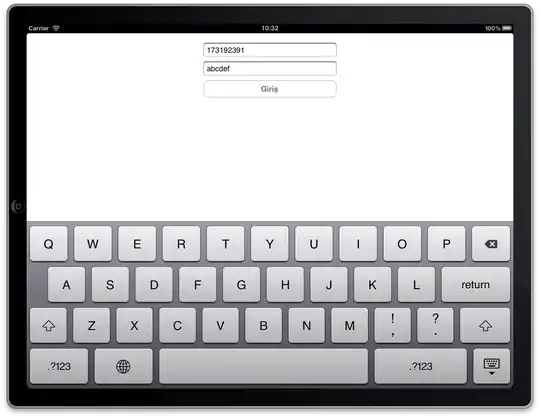
As you now know the 3 sides of triangle (chord length,radius(other 2 sides) you calculate bearing of center of arc using law of cosines.(Note there are 2 solutions as discuused in comments)
function solveAngle(a, b, c) { // Returns angle C using law of cosines
var temp = (b * b + c * c - a * a) / (2 * b * c);
if (temp >= -1 && temp <= 1)
return Math.acos(temp);
else
throw "No solution";
}
var baseAngle = solveAngle(radius, radius, c);
var vertexAngle = solveAngle(c,radius,radius);
baseAngle
is used to find bearing of center point.
vertexAngle
is used number of points when drawing arc.
Knowing bearing and radius you can find center of arc.
var centerPoint = spherical.computeOffset(point1,radius,heading+baseAngle);
Note Distances in meters. change radius in methods to 3,959 for miles.
If the radius changes the center changes. The yellow dot is 2 times the radius of blue dot
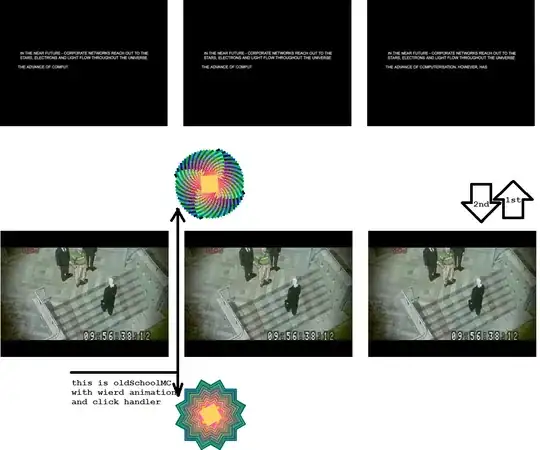