I had my own fix and it's not one click, but I found it well worth it.
Step 1:
Test some font sizes for different screen sizes, recording the font size and the most relevant other dimension.
Here's what I mean...
I'd start with a normal iPad Screen and select a font size that worked.

Since I knew the entire height of the screen would determine what makes a font size comfortable or not, I'd record the font size along with the entire screen height.
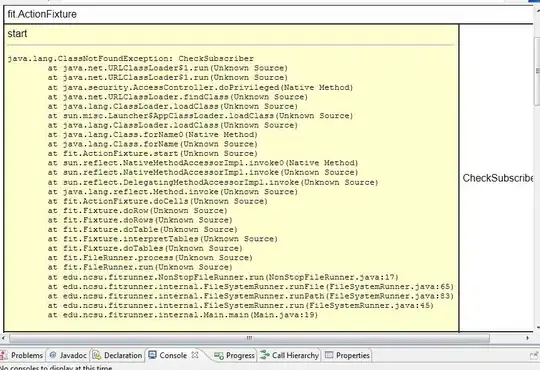
So the font size was 50 with a height of 1024.
Then, I switched to the iPhone SE
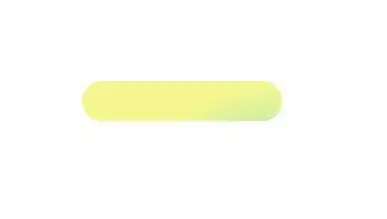
This was pretty atrocious, so I fixed the font size:

After recording that the font size worked at 25 with a height of 568.
Step 2:
Bust out the calculator app and find a constant that relates the font sizes to the heights (in my case), giving or taking a bit.
Here's what I mean:
I knew 50 works with 1024 and that 25 works with 568.
Dividing 50/1024 gives you .048828125.
Multiplying that by 568 equals 27.734375, which would be a little fat of a font size for the SE.
Repeating that process but using the SE's values to do the division produced .0440140845, and checking that with the iPad's height produces a font size of 45, just a bit small for the mighty iPad screen.
So I split the quotients down the middle, resulting in a number around 0.46.
Step 3:
Connect the labels and change their font sizes programmatically using this number.
First, drag the labels into an outlet collection. I named my connection 'headerLabels' since they're all at the top of the screen.
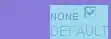
Then, you can kinda steal my code, but just don't make too much money with it ;)
// Outlet collection of labels
@IBOutlet var headerLabels: [UILabel]!
// Who needs a short variable name when we can have a
// painfully lengthy one to describe the number we calculated?
let relativeFontConstant:CGFloat = 0.046
override func viewDidLoad() {
super.viewDidLoad()
// Should be in the viewDidLoad so it can load the same time as
// the view does.
// apply a font size to all the labels.
for label in headerLabels {
// multiply the height we based it on of the entire view
// to the number we calculated
label.font = label.font.withSize(self.view.frame.height * relativeFontConstant)
}
}
If you've got any questions, comments, compliments, or insults let me know :)