There's no need to use a third-party library since Google introduced the TextInputLayout
as part of the design-support-library
.
Following a basic example:
Layout
<android.support.design.widget.TextInputLayout
android:id="@+id/text_input_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:errorEnabled="true">
<android.support.design.widget.TextInputEditText
android:id="@+id/edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter your name" />
</android.support.design.widget.TextInputLayout>
Note: By setting app:errorEnabled="true"
as an attribute of the TextInputLayout
it won't change it's size once an error is displayed - so it basically blocks the space.
Code
In order to show the Error below the EditText
you simply need to call #setError
on the TextInputLayout
(NOT on the child EditText
):
TextInputLayout til = (TextInputLayout) findViewById(R.id.text_input_layout);
til.setError("You need to enter a name");
Result
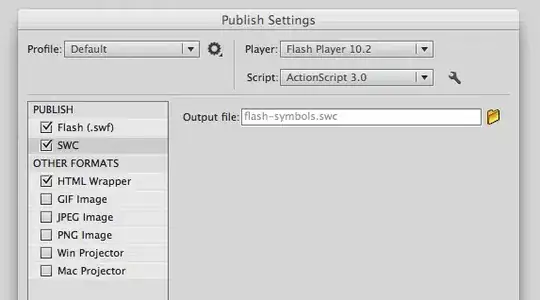
To hide the error and reset the tint simply call til.setError(null)
.
Note
In order to use the TextInputLayout
you have to add the following to your build.gradle
dependencies:
dependencies {
compile 'com.android.support:design:25.1.0'
}
Setting a custom color
By default the line of the EditText
will be red. If you need to display a different color you can use the following code as soon as you call setError
.
editText.getBackground().setColorFilter(getResources().getColor(R.color.red_500_primary), PorterDuff.Mode.SRC_ATOP);
To clear it simply call the clearColorFilter
function, like this:
editText.getBackground().clearColorFilter();