You can simply draw the checkmark in the Paint event:

private void checkBox1_Paint(object sender, PaintEventArgs e)
{
Point pt = new Point(e.ClipRectangle.Left + 2, e.ClipRectangle.Top + 4);
Rectangle rect = new Rectangle(pt, new Size(22, 22));
if (checkBox1.Checked)
{
using (Font wing = new Font("Wingdings", 14f))
e.Graphics.DrawString("ü", wing, Brushes.DarkOrange,rect);
}
e.Graphics.DrawRectangle(Pens.DarkSlateBlue, rect);
}
for this to work you need to:
- set
Apperance = Appearance.Button
- set
FlatStyle = FlatStyle.Flat
- set
TextAlign = ContentAlignment.MiddleRight
- set
FlatAppearance.BorderSize = 0
- set
AutoSize = false
If you want to re-use this it will be best to subclass the checkbox and override the OnPaint
event there. Here is an example:
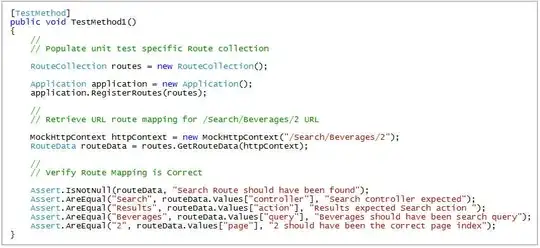
public ColorCheckBox()
{
Appearance = System.Windows.Forms.Appearance.Button;
FlatStyle = System.Windows.Forms.FlatStyle.Flat;
TextAlign = ContentAlignment.MiddleRight;
FlatAppearance.BorderSize = 0;
AutoSize = false;
Height = 16;
}
protected override void OnPaint(PaintEventArgs pevent)
{
//base.OnPaint(pevent);
pevent.Graphics.Clear(BackColor);
using (SolidBrush brush = new SolidBrush(ForeColor))
pevent.Graphics.DrawString(Text, Font, brush, 27, 4);
Point pt = new Point( 4 , 4);
Rectangle rect = new Rectangle(pt, new Size(16, 16));
pevent.Graphics.FillRectangle(Brushes.Beige, rect);
if (Checked)
{
using (SolidBrush brush = new SolidBrush(ccol))
using (Font wing = new Font("Wingdings", 12f))
pevent.Graphics.DrawString("ü", wing, brush, 1,2);
}
pevent.Graphics.DrawRectangle(Pens.DarkSlateBlue, rect);
Rectangle fRect = ClientRectangle;
if (Focused)
{
fRect.Inflate(-1, -1);
using (Pen pen = new Pen(Brushes.Gray) { DashStyle = DashStyle.Dot })
pevent.Graphics.DrawRectangle(pen, fRect);
}
}
You may need to tweek the sizes of the control and the font.. And if you want to you expand the code to honor the TextAlign
and the CheckAlign
properties.
And if you need a three-state control you can adapt the code to display a third state appearance, especially if you think of one that looks better than the original..