To generate some text we can use any method, here I am using ImageFont to get a black and white mask.
def generate_text(text):
fnt = ImageFont.truetype('arial.ttf', 72)
img = Image.new('1', fnt.getsize(text))
mask = [x for x in fnt.getmask(text, mode='1')]
img.putdata(mask)
img = img.convert('RGB')
return img
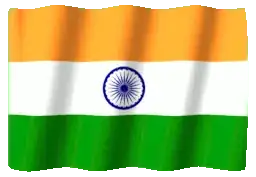
Then, I would like to add padding on the left and right because we will be shearing the text horizontally.
def add_border(img, width):
new = Image.new('RGB', (img.width + 2 * width, img.height), (0, 0, 0))
new.paste(img, (width, 0))
return new
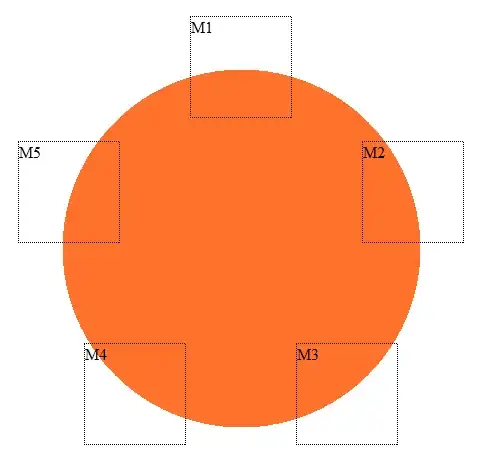
Finally, I will use an affine transformation using the b
value, which if you look a the formula, it is the coefficient of y
that will be added to x
. So, a larger b
will shear horizontally more. In this case I am using 2
.
def shear(img, shear):
shear = img.transform(img.size, Image.AFFINE, (1, shear, 0, 0, 1, 0))
return shear
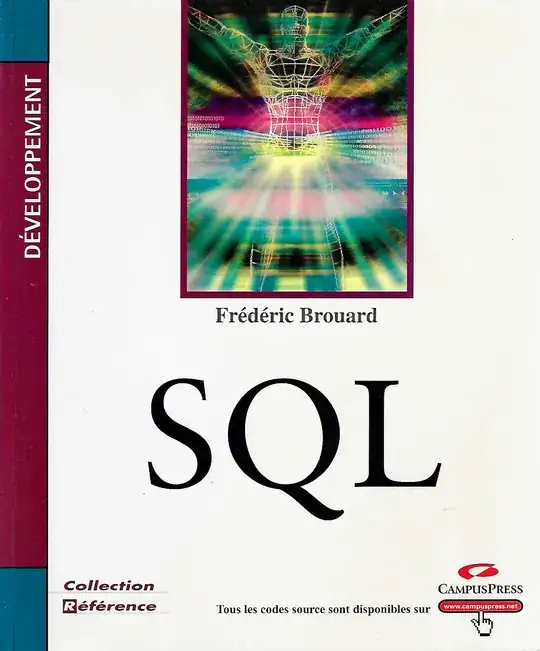
The calling code:
img = generate_text('test')
img = add_border(img, 200)
img = shear(img, 2)