The problem can be reduced to the intersection of an ellipse and a circle (you can scale 1 dimension). However, there doesn't seem to be a short answer. See Ellipse–circle and ellipse–ellipse collision detection and How to detect if an ellipse intersects(collides with) a circle. The major issue is to find the closest point on the ellipse to the circle.
Even though I don't think the question here was about a collision masks, but about finding a formula that is more powerful than using masks where both masks have to be passed through and the mask bits compared, I will provide a complete alternative solution using masks:
mask1 = pygame.mask.from_surface(ellipse_surf1)
mask2 = pygame.mask.from_surface(ellipse_surf2)
offset_x = ellipse_rect2.x - ellipse_rect1.x
offset_y = ellipse_rect2.y - ellipse_rect2.y
overlap_mask = mask1.overlap_mask(mask2, (offset_x, offset_y))
Complete example:
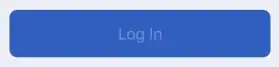
import pygame
def create_ellipse_angle(color, rect, angle, width=0):
target_rect = pygame.Rect(rect)
shape_surf = pygame.Surface(target_rect.size, pygame.SRCALPHA)
pygame.draw.ellipse(shape_surf, color, (0, 0, *target_rect.size), width)
rotated_surf = pygame.transform.rotate(shape_surf, angle)
bounding_rect = rotated_surf.get_rect(center = target_rect.center)
return rotated_surf, bounding_rect
pygame.init()
window = pygame.display.set_mode((400, 250))
clock = pygame.time.Clock()
background = pygame.Surface(window.get_size())
ts, w, h, c1, c2 = 50, *window.get_size(), (160, 160, 160), (192, 192, 192)
tiles = [((x*ts, y*ts, ts, ts), c1 if (x+y) % 2 == 0 else c2) for x in range((w+ts-1)//ts) for y in range((h+ts-1)//ts)]
for rect, color in tiles:
pygame.draw.rect(background, color, rect)
angle1 = 0
angle2 = 0
run = True
while run:
clock.tick(60)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
ellipse_surf1, ellipse_rect1 = create_ellipse_angle((0, 128, 128), (35, 75, 200, 100), angle1)
angle1 += 1
ellipse_surf2, ellipse_rect2 = create_ellipse_angle((128, 0, 128), (165, 75, 200, 100), angle2)
angle2 -= 0.5
mask1 = pygame.mask.from_surface(ellipse_surf1)
mask2 = pygame.mask.from_surface(ellipse_surf2)
offset_x = ellipse_rect2.x - ellipse_rect1.x
offset_y = ellipse_rect2.y - ellipse_rect2.y
overlap_mask = mask1.overlap_mask(mask2, (offset_x, offset_y))
overlap_surf = overlap_mask.to_surface(setcolor = (255, 0, 0))
overlap_surf.set_colorkey((0, 0, 0))
window.blit(background, (0, 0))
window.blit(ellipse_surf1, ellipse_rect1)
window.blit(ellipse_surf2, ellipse_rect2)
window.blit(overlap_surf, ellipse_rect1)
pygame.display.flip()
pygame.quit()
exit()