I also had this issue with my Android app depending on some of my own Android libraries (using Android Studio 3.0 and 3.1.1).
Whenever I updated a lib and go back to the app, triggering a Gradle Sync, Android Studio was not able to detect the code changes I made to the lib. Compilation worked fine, but Android Studio showed red error lines on some code using the lib.
After investigating, I found that it's because gradle keeps pointing to an old compiled version of my libs.
If you go to yourProject/.idea/libraries/ you'll see a list of xml files that contains the link to the compiled version of your libs. These files starts with Gradle__artifacts_*.xml (where * is the name of your libs).
So in order for Android Studio to take the latest version of your libs, you need to delete these Gradle__artifacts_*.xml files, and Android Studio will regenerate them, pointing to the latest compiled version of your libs.
If you don't want to do that manually every time you click on "Gradle sync" (who would want to do that...), you can add this small gradle task in the build.gradle file of your app.
task deleteArtifacts {
doFirst {
File librariesFolderPath = file(getProjectDir().absolutePath + "/../.idea/libraries/")
File[] files = librariesFolderPath.listFiles({ File file -> file.name.startsWith("Gradle__artifacts_") } as FileFilter)
for (int i = 0; i < files.length; i++) {
files[i].delete()
}
}
}
And in order for your app to always execute this task before doing a gradle sync, you just need to go to the Gradle window, then find the "deleteArtifacts" task under yourApp/Tasks/other/, right click on it and select "Execute Before Sync" (see below).
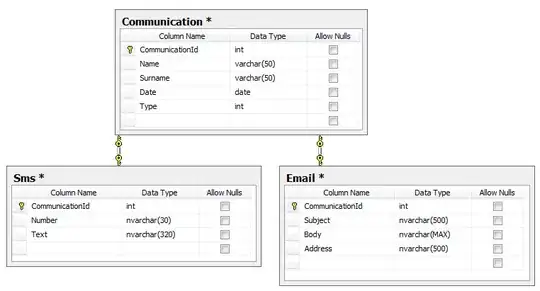
Now, every time you do a Gradle sync, Android Studio will be forced to use the latest version of your libs.